Python Math: Get the sum of the powers of all the numbers from start to end (both inclusive)
85. Sum of Powers Calculator
Write a Python program to get the sum of the powers of all the numbers from start to end (both inclusive).
- Use range() in combination with a list comprehension to create a list of elements in the desired range raised to the given power.
- Use sum() to add the values together.
- Omit the second argument, power, to use a default power of 2.
- Omit the third argument, start, to use a default starting value of 1.
Sample Solution:
Python Code:
def sum_of_powers(end, power = 2, start = 1):
return sum([(i) ** power for i in range(start, end + 1)])
print(sum_of_powers(12))
print(sum_of_powers(12, 3))
print(sum_of_powers(12, 5, 7))
Sample Output:
650 6084 618507
Flowchart:
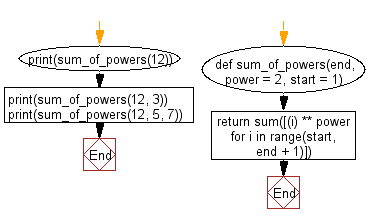
For more Practice: Solve these Related Problems:
- Write a Python program to calculate the sum of numbers raised to a given power from a start value to an end value, then print the sum.
- Write a Python function that accepts parameters start, end, and power, computes the sum of the numbers raised to that power, and returns the result.
- Write a Python script to compare the sum of powers for different exponents over the same range and print a summary of the results.
- Write a Python program to prompt the user for a start value, end value, and exponent, then calculate and display the sum of the powers.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to get the nth tetrahedral number from a given integer(n) value.
Next: Write a Python program to calculate the Hamming distance between two given values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.