Python Math: Create a Pythagorean theorem calculator
68. Pythagorean Theorem Calculator
Write a Python program to create a Pythagorean theorem calculator.
Note : In mathematics, the Pythagorean theorem, also known as Pythagoras' theorem, is a fundamental relation in Euclidean geometry among the three sides of a right triangle. It states that the square of the hypotenuse (the side opposite the right angle) is equal to the sum of the squares of the other two sides.
Sample Solution:
Python Code:
from math import sqrt
print('Pythagorean theorem calculator! Calculate your triangle sides.')
print('Assume the sides are a, b, c and c is the hypotenuse (the side opposite the right angle')
formula = input('Which side (a, b, c) do you wish to calculate? side> ')
if formula == 'c':
side_a = int(input('Input the length of side a: '))
side_b = int(input('Input the length of side b: '))
side_c = sqrt(side_a * side_a + side_b * side_b)
print('The length of side c is: ' )
print(side_c)
elif formula == 'a':
side_b = int(input('Input the length of side b: '))
side_c = int(input('Input the length of side c: '))
side_a = sqrt((side_c * side_c) - (side_b * side_b))
print('The length of side a is' )
print(side_a)
elif formula == 'b':
side_a = int(input('Input the length of side a: '))
side_b = int(input('Input the length of side c: '))
side_c = sqrt(side_c * side_c - side_a * side_a)
print('The length of side b is')
print(side_c)
else:
print('Please select a side between a, b, c')
Sample Output:
Pythagorean theorem calculator! Calculate your triangle sides. Assume the sides are a, b, c and c is the hypotenuse (the side opposite the right angle Which side (a, b, c) do you wish to calculate? side>a Input the length of side b:10 Input the length of side c:15 The length of side a is 11.180339887498949
Flowchart:
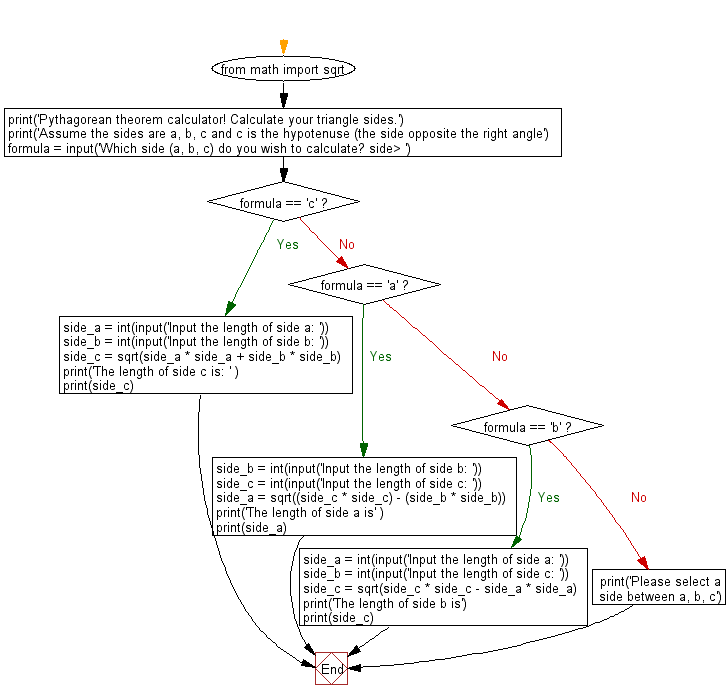
For more Practice: Solve these Related Problems:
- Write a Python program to implement the Pythagorean theorem for a right triangle and calculate the missing side based on user input.
- Write a Python function that takes two sides of a right triangle as input and returns the hypotenuse using the Pythagorean theorem.
- Write a Python script to compare the hypotenuse calculated using the Pythagorean theorem with the result from math.hypot().
- Write a Python program to prompt the user for two sides of a right triangle, compute the missing side, and then display the result with 4 decimal precision.
Go to:
Previous: Write a Python program to create a dot string.
Next: Write a Python function to round up a number to specified digits.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.