Python Math: Find the roots of a quadratic function
Python Math: Exercise-30 with Solution
Write a Python program to find the roots of a quadratic function.
Sample Solution:
Python Code:
from math import sqrt
print("Quadratic function : (a * x^2) + b*x + c")
a = float(input("a: "))
b = float(input("b: "))
c = float(input("c: "))
r = b**2 - 4*a*c
if r > 0:
num_roots = 2
x1 = (((-b) + sqrt(r))/(2*a))
x2 = (((-b) - sqrt(r))/(2*a))
print("There are 2 roots: %f and %f" % (x1, x2))
elif r == 0:
num_roots = 1
x = (-b) / 2*a
print("There is one root: ", x)
else:
num_roots = 0
print("No roots, discriminant < 0.")
exit()
Sample Output:
Quadratic function : (a * x^2) + b*x + c a: 5 b: 20 c: 10 There are 2 roots: -0.585786 and -3.414214
Flowchart:
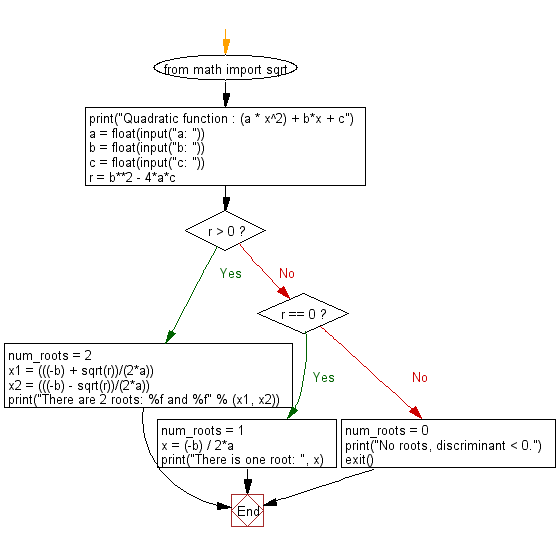
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to calculate wind chill index.
Next: Write a Python program to convert a binary number to decimal number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/math/python-math-exercise-30.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics