Python Math: Multiply two integers without using the * operator in python
19. Multiply Without Operator
Write a Python program to multiply two integers without using the * operator.
Sample Solution:
Python Code:
def multiply(x, y):
if y < 0:
return -multiply(x, -y)
elif y == 0:
return 0
elif y == 1:
return x
else:
return x + multiply(x, y - 1)
print(multiply(3, 5));
Sample Output:
15
Flowchart:
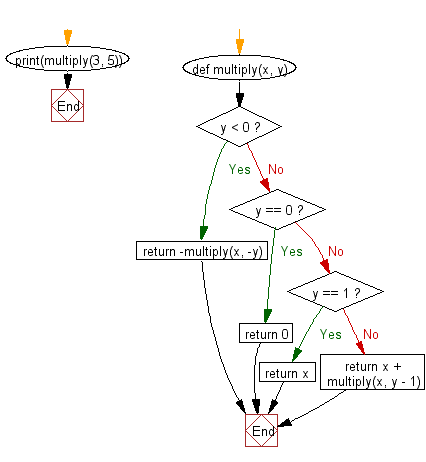
For more Practice: Solve these Related Problems:
- Write a Python program to multiply two integers using only addition and bit shifting, and then print the product.
- Write a Python function that multiplies two numbers recursively without using the * operator, then test it with several inputs.
- Write a Python script to implement an iterative multiplication algorithm (using loops) without *, and compare the result with the built-in multiplication.
- Write a Python program to simulate multiplication using repeated addition and print the intermediate sums leading to the final product.
Go to:
Previous: Write a Python program to computing square roots using the Babylonian method.
Next: Write a Python program to calculate magic square.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.