Python Math: Convert degree to radian
1. Degrees to Radians Conversion
Write a Python program to convert degrees to radians.
Note : The radian is the standard unit of angular measure, used in many areas of mathematics. An angle's measurement in radians is numerically equal to the length of a corresponding arc of a unit circle; one radian is just under 57.3 degrees (when the arc length is equal to the radius).
Test Data:
Degree : 15
Expected Result in radians: 0.2619047619047619
Sample Solution-1:
Python Code:
pi=22/7
degree = float(input("Input degrees: "))
radian = degree*(pi/180)
print(radian)
Sample Output:
Input degrees: 90 1.5714285714285714
Pictorial Presentation:
Flowchart:
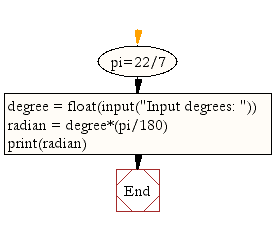
Sample Solution-2:
Use math.pi and the degrees to radians formula to convert the angle from degrees to radians.
Python Code:
from math import pi
def degrees_to_rads(deg):
return (deg * pi) / 180.0
print(degrees_to_rads(180))
print(degrees_to_rads(90))
Sample Output:
3.141592653589793 1.5707963267948966
Flowchart:
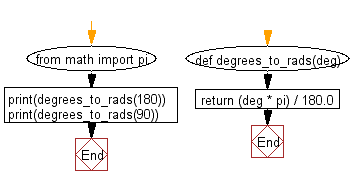
Visualize Python code execution:
The following tool visualize what the computer is doing step-by-step as it executes the said program:
For more Practice: Solve these Related Problems:
- Write a Python function that accepts an angle in degrees, converts it to radians using math.radians(), and returns the result rounded to 10 decimal places.
- Write a Python program that reads a list of degree values from the user, converts each to radians, and prints both the original and converted values in a formatted table.
- Write a Python script that compares the output of your own degrees-to-radians conversion function with math.radians() for a series of test angles.
- Write a Python function that converts degrees to radians without using math.radians() (i.e. by multiplying by π/180) and prints the conversion for 15°.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Python Math Exercise Home.
Next: Write a Python program to convert radian to degree.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.