Python: Round every number of a given list of numbers and print the total sum multiplied by the length of the list
Round Numbers and Calculate Total Sum
Write a Python program to round every number in a given list of numbers and print the total sum multiplied by the length of the list.
Visual Presentation:
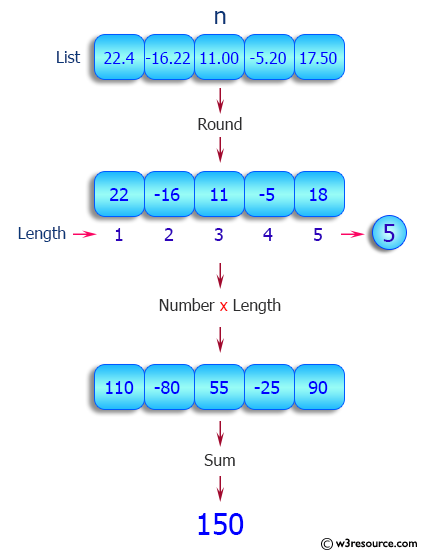
Sample Solution:
Python Code:
# Create a list 'nums' containing floating-point numbers
nums = [22.4, 4.0, -16.22, -9.10, 11.00, -12.22, 14.20, -5.20, 17.50]
# Print a message indicating the original list
print("Original list: ", nums)
# Print a message indicating the result
print("Result:")
# Calculate the length of the 'nums' list and store it in the 'lenght' variable
lenght = len(nums)
# Calculate the sum of the rounded values in 'nums' using the map function,
# and then multiply the sum by the length of the list 'lenght'
print(sum(list(map(round, nums)) * lenght))
Sample Output:
Original list: [22.4, 4.0, -16.22, -9.1, 11.0, -12.22, 14.2, -5.2, 17.5] Result: 243
Flowchart:
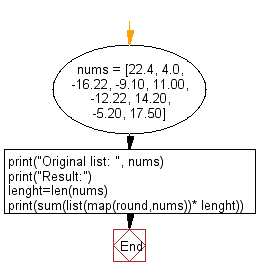
Python Code Editor:
Previous: Write a Python program to generate the combinations of n distinct objects taken from the elements of a given list.
Next: Write a Python program to round the numbers of a given list, print the minimum and maximum numbers and multiply the numbers by 5. Print the unique numbers in ascending order separated by space.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics