Python: Remove the K'th element from a given list, print the new list
Remove K-th Element from List
Write a Python program to remove the K'th element from a given list, and print the updated list.
Sample Solution:
Python Code:
# Define a function 'remove_kth_element' that takes a list 'n_list' and an integer 'L' as input
def remove_kth_element(n_list, L):
# Return a modified list by removing the element at the kth position (L-1) from the input list
return n_list[:L - 1] + n_list[L:]
# Create a list 'n_list' containing integers
n_list = [1, 1, 2, 3, 4, 4, 5, 1]
# Print a message indicating the original list
print("Original list:")
# Print the original list
print(n_list)
# Assign an integer 'kth_position' with the value 3
kth_position = 3
# Call the 'remove_kth_element' function with 'n_list' and 'kth_position'
# and store the result in the 'result' variable
result = remove_kth_element(n_list, kth_position)
# Print a message indicating the list after removing an element at the kth position
print("\nAfter removing an element at the kth position of the said list:")
# Print the 'result' list
print(result)
Sample Output:
Original list: [1, 1, 2, 3, 4, 4, 5, 1] After removing an element at the kth position of the said list: [1, 1, 3, 4, 4, 5, 1]
Flowchart:
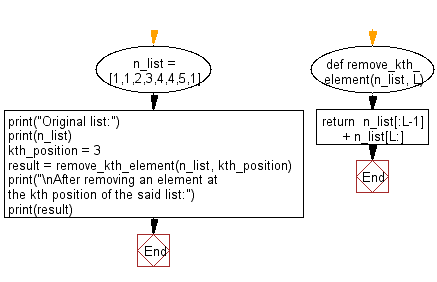
For more Practice: Solve these Related Problems:
- Write a Python program to remove multiple elements at specified indices from a list.
- Write a Python program to remove every K-th element from a list in a cyclic manner.
- Write a Python program to remove the K-th element from a list without using loops.
- Write a Python program to remove K-th elements from a list and store them separately.
Go to:
Previous: Write a Python program to split a given list into two parts where the length of the first part of the list is given.
Next: Write a Python program to insert an element at a specified position into a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.