Python: Create a list reflecting the run-length encoding from a list
Create Run-Length Encoded List
Run-length encoding (RLE) is a form of lossless data compression in which runs of data (sequences in which the same data value occurs in many consecutive data elements) are stored as a single data value and count, rather than as the original run.
Write a Python program to create a list reflecting the run-length encoding from a given list of integers or a given list of characters.
Sample Solution:
Python Code:
# Import the 'groupby' function from the 'itertools' module
from itertools import groupby
# Define a function 'encode_list' that takes a list 's_list' as input
def encode_list(s_list):
# Use 'groupby' to group consecutive elements and count their occurrences
return [[len(list(group)), key] for key, group in groupby(s_list)]
# Define a list 'n_list' with various elements including duplicates
n_list = [1, 1, 2, 3, 4, 4.3, 5, 1]
# Print a message indicating the purpose of the following output
print("Original list:")
# Print the original list 'n_list'
print(n_list)
# Print a message indicating the purpose of the following output
print("\nList reflecting the run-length encoding from the said list:")
# Call the 'encode_list' function with 'n_list' as an argument and print the result of run-length encoding
print(encode_list(n_list))
# Reassign 'n_list' with a string
n_list = 'automatically'
# Print a message indicating the purpose of the following output
print("\nOriginal String:")
# Print the original string 'n_list'
print(n_list)
# Print a message indicating the purpose of the following output
print("\nList reflecting the run-length encoding from the said string:")
# Call the 'encode_list' function with 'n_list' as an argument and print the result of run-length encoding for the string
print(encode_list(n_list))
Sample Output:
Original list: [1, 1, 2, 3, 4, 4.3, 5, 1] List reflecting the run-length encoding from the said list: [[2, 1], [1, 2], [1, 3], [1, 4], [1, 4.3], [1, 5], [1, 1]] Original String: automatically List reflecting the run-length encoding from the said string: [[1, 'a'], [1, 'u'], [1, 't'], [1, 'o'], [1, 'm'], [1, 'a'], [1, 't'], [1, 'i'], [1, 'c'], [1, 'a'], [2, 'l'], [1, 'y']]
Flowchart:
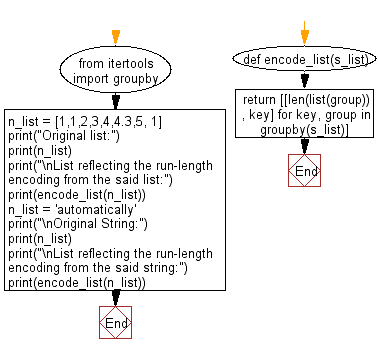
For more Practice: Solve these Related Problems:
- Write a Python program to implement run-length encoding for a string.
- Write a Python program to decode a run-length encoded list back to the original list.
- Write a Python program to perform run-length encoding, but group elements into tuples.
- Write a Python program to find the most frequently occurring element using run-length encoding.
Go to:
Previous: Write a Python program to pack consecutive duplicates of a given list elements into sublists.
Next: Write a Python program to create a list reflecting the modified run-length encoding from a given list of integers or a given list of characters.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.