Python: Get the depth of a dictionary
Python Dictionary: Exercise - 54 with Solution
Write a Python program to get the depth of a dictionary.
Sample Solution:
Python Code:
def dict_depth(d):
if isinstance(d, dict):
return 1 + (max(map(dict_depth, d.values())) if d else 0)
return 0
dic = {'a':1, 'b': {'c': {'d': {}}}}
print(dict_depth(dic))
Sample Output:
4
Flowchart:
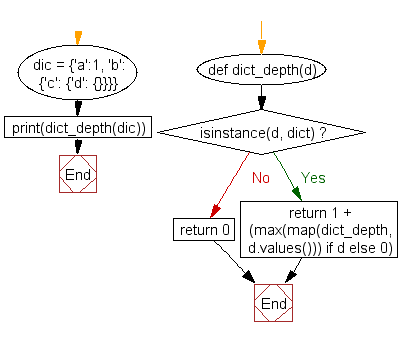
Python Code Editor:
Previous: Write a Python program to find the length of a given dictionary values.
Next: Write a Python program to access dictionary key’s element by index.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/list/python-data-type-list-exercise-70.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics