Python: Count the number of strings where the string length is 2 or more and the first and last character are same from a given list of strings
Count Strings with Same Start and End
Write a Python program to count the number of strings from a given list of strings. The string length is 2 or more and the first and last characters are the same.
Sample Solution:
Python Code:
# Define a function called match_words that takes a list of words 'words' as input
def match_words(words):
# Initialize a counter 'ctr' to keep track of matching words
ctr = 0
# Iterate through each word in the input list 'words'
for word in words:
# Check if the word has a length greater than 1 and its first and last characters are the same
if len(word) > 1 and word[0] == word[-1]:
# If the condition is met, increment the counter 'ctr'
ctr += 1
# Return the final count of matching words
return ctr
# Call the match_words function with the list ['abc', 'xyz', 'aba', '1221'] as input and print the result
print(match_words(['abc', 'xyz', 'aba', '1221']))
Sample Output:
2
Explanation:
In the above exercise -
def match_words(words): -> Defines a function called ‘match_words’ that takes a single argument words. This function will count the number of words in the list that have the same first and last letter.
ctr = 0 -> This line initializes a variable called ctr to 0. This variable will be used to keep track of the number of words in the list that match the criteria.
for word in words: -> This line starts a loop that will iterate over each element in the words list, one at a time. The loop variable word will take on the value of each element in the list during each iteration of the loop.
if len(word) > 1 and word[0] == word[-1]: -> This line checks if the length of the current word is greater than 1 and if the first letter of the word is the same as the last letter of the word. If these conditions are both true, it means the word has the same first and last letter.
ctr += 1 -> This line increments the 'ctr' variable by 1 if the current word meets the criteria.
return ctr -> This line returns the final value of the 'ctr' variable after the loop has finished.
print(match_words(['abc', 'xyz', 'aba', '1221'])) -> This line calls the ‘match_words’ function and passes in the list ['abc', 'xyz', 'aba', '1221']. The final output will be 2.
Flowchart:
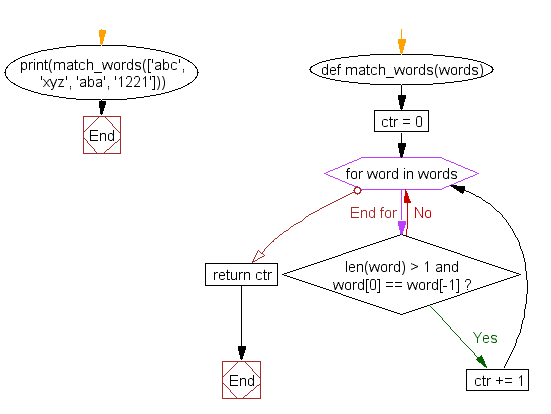
Python Code Editor:
Previous: Write a Python program to get the smallest number from a list.
Next: Write a Python program to get a list, sorted in increasing order by the last element in each tuple from a given list of non-empty tuples.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics