Python: Convert a pair of values into a sorted unique array
Python List: Exercise - 45 with Solution
Write a Python program to convert a pair of values into a sorted unique array.
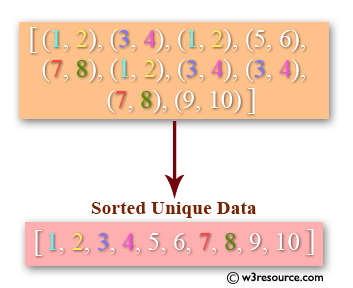
Sample Solution:
Python Code:
# Define a list 'L' containing tuples with pairs of numbers
L = [(1, 2), (3, 4), (1, 2), (5, 6), (7, 8), (1, 2), (3, 4), (3, 4), (7, 8), (9, 10)]
# Print the original list 'L'
print("Original List: ", L)
# Use a set to remove duplicate pairs of numbers and the 'union' method to merge all unique pairs
# Sort the resulting set and print the sorted unique data
print("Sorted Unique Data:", sorted(set().union(*L)))
Sample Output:
Original List: [(1, 2), (3, 4), (1, 2), (5, 6), (7, 8), (1, 2), (3, 4), (3, 4), (7, 8), (9, 10)] Sorted Unique Data: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Flowchart:
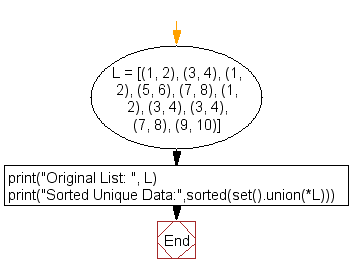
Python Code Editor:
Previous: Write a Python program to generate groups of five consecutive numbers in a list.
Next: Write a Python program to select the odd items of a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/list/python-data-type-list-exercise-45.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics