Python Exercises: Check if each number is prime in a list of numbers
Extract Specified Vowels
Write a Python program to check if each number is prime in a given list of numbers. Return True if all numbers are prime otherwise False.
Sample Data:
([0, 3, 4, 7, 9]) -> False
([3, 5, 7, 13]) -> True
([1, 5, 3]) -> False
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes two inputs: 'text' (a string) and 'n' (an integer).
def test(text, n):
result_str = '' # Initialize an empty string to store the vowels.
for i in text:
# Iterate through each character 'i' in the 'text' string.
if i in 'aioueAEIOU':
# Check if the current character 'i' is a vowel (lowercase or uppercase).
result_str += i # If it's a vowel, append it to 'result_str'.
# Check if the length of 'result_str' is greater than or equal to 'n'.
if len(result_str) >= n:
# If it is, return the first 'n' characters of 'result_str', which are the vowels.
return result_str[:n]
else:
# If 'n' is greater than the number of vowels, return a message indicating so.
return 'n is less than the number of vowels present in the string.'
# Define the original string 'word' and the number 'n'.
word = "Python"
n = 2
print("Original string and number:", word, ",", n)
# Call the 'test' function to extract the first 'n' vowels from the string 'word'.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define another string 'word' and a different number 'n'.
word = "Python Exercises"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the new inputs to extract the first 'n' vowels from the string.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define a string 'word' consisting of only vowels and a different number 'n'.
word = "AEIOU"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the vowel-only string and 'n' to extract the first 'n' vowels.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
Sample Output:
Original list of numbers: [0, 3, 4, 7, 9] Check if each number is prime in the said list of numbers: False Original list of numbers: [3, 5, 7, 13] Check if each number is prime in the said list of numbers: True Original list of numbers: [1, 5, 3] Check if each number is prime in the said list of numbers: False
Flowchart:
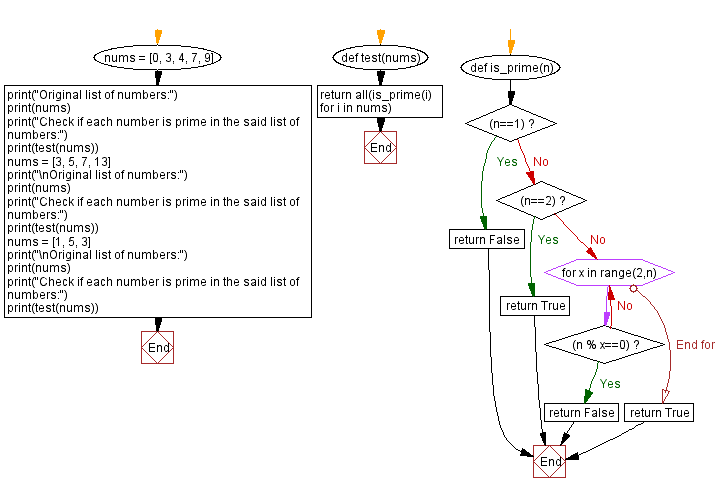
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes two inputs: 'text' (a string) and 'n' (an integer).
def test(text, n):
# Use a list comprehension to create a list 't' containing characters from 'text' that are vowels (lowercase or uppercase).
t = [x for x in text if x in 'aeiouAEIOU']
# Check if the length of the 't' list is less than 'n'.
if len(t) < n:
# If 'n' is greater than the number of vowels in 'text', return a message indicating so.
return 'n is less than the number of vowels present in the string.'
else:
# If there are enough vowels, join the first 'n' vowels from 't' into a string and return it.
return ''.join(t[:n])
# Define the original string 'word' and the number 'n'.
word = "Python"
n = 2
print("Original string and number:", word, ",", n)
# Call the 'test' function to extract the first 'n' vowels from the string 'word'.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define another string 'word' and a different number 'n'.
word = "Python Exercises"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the new inputs to extract the first 'n' vowels from the string.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
# Define a string 'word' consisting of only vowels and a different number 'n'.
word = "AEIOU"
n = 3
print("\nOriginal string and number:", word, ",", n)
# Call the 'test' function with the vowel-only string and 'n' to extract the first 'n' vowels.
print("Extract the first n number of vowels from the said string:")
print(test(word, n))
Sample Output:
Original list of numbers: [0, 3, 4, 7, 9] Check if each number is prime in the said list of numbers: False Original list of numbers: [3, 5, 7, 13] Check if each number is prime in the said list of numbers: True Original list of numbers: [1, 5, 3] Check if each number is prime in the said list of numbers: False
Flowchart:
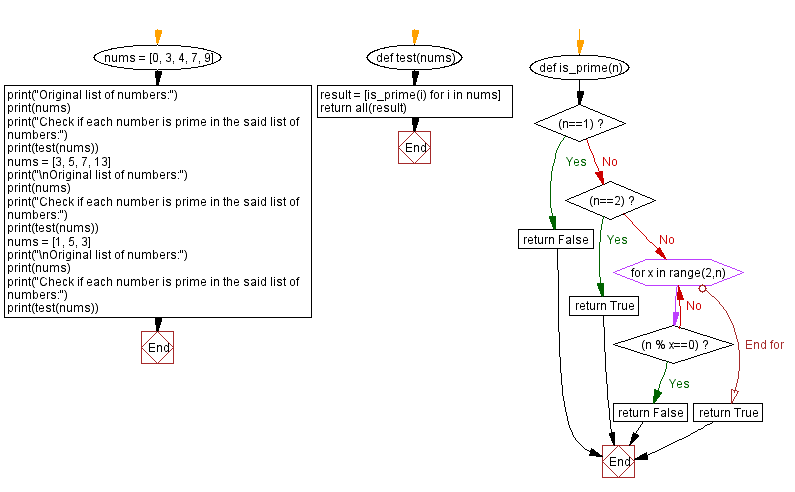
For more Practice: Solve these Related Problems:
- Write a Python program to extract the first n vowels from a string, ignoring case, and return them as uppercase.
- Write a Python program to extract all vowels from a string and then return only the first n unique vowels.
- Write a Python program to count the vowels in a string and extract them only if their count exceeds a given threshold.
- Write a Python program to extract the last n vowels from a string and reverse their order.
Python Code Editor:
Previous Python Exercise: Sum of missing numbers of a list of integers.
Next Python Exercise: Extract the first n number of vowels from a string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.