Python: Find the powerset of a given iterable
Generate Powerset of Iterable
Write a Python program to get the powerset of a given iterable.
Note: A Power Set is a set of all the subsets of a set.
- Use list() to convert the given value to a list.
- Use range() and itertools.combinations() to create a generator that returns all subsets.
- Use itertools.chain.from_iterable() and list() to consume the generator and return a list.
Sample Solution:
Python Code:
from itertools import chain, combinations
# Define a function to generate the powerset of an iterable.
def powerset(iterable):
s = list(iterable) # Convert the input iterable to a list.
return list(chain.from_iterable(combinations(s, r) for r in range(len(s) + 1)))
# Example 1
nums = [1, 2]
print("Original list elements:")
print(nums)
print("Powerset of the said list:")
print(powerset(nums))
# Example 2
nums = [1, 2, 3, 4]
print("\nOriginal list elements:")
print(nums)
print("Powerset of the said list:")
print(powerset(nums))
Sample Output:
Original list elements: [1, 2] Powerset of the said list: [(), (1,), (2,), (1, 2)] Original list elements: [1, 2, 3, 4] Powerset of the said list: [(), (1,), (2,), (3,), (4,), (1, 2), (1, 3), (1, 4), (2, 3), (2, 4), (3, 4), (1, 2, 3), (1, 2, 4), (1, 3, 4), (2, 3, 4), (1, 2, 3, 4)]
Flowchart:
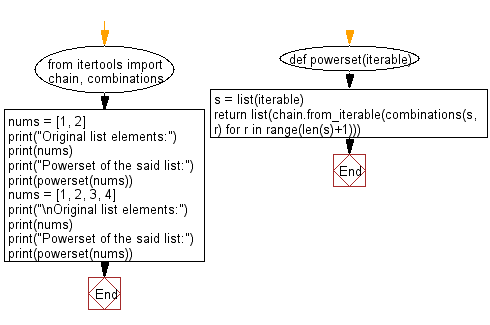
For more Practice: Solve these Related Problems:
- Write a Python program to generate the powerset of a given iterable excluding the empty set.
- Write a Python program to compute the powerset of a list and then filter out subsets that do not meet a specified size criterion.
- Write a Python program to generate the powerset of an iterable and return the result as a list of sorted tuples.
- Write a Python program to generate the powerset of a list and then calculate the sum of elements for each subset.
Go to:
Previous: Write a Python program to perform a deep flattens a list.
Next: Write a Python program to check if two given lists contain the same elements regardless of order.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.