Python: Convert a list of dictionaries into a list of values corresponding to the specified key
Extract Values by Key
Write a Python program to convert a given list of dictionaries into a list of values corresponding to the specified key.
Use a list comprehension and dict.get() to get the value of key for each dictionary in lst.
Sample Solution:
Python Code:
# Define a function 'pluck' that takes a list 'lst' and a key 'key' as input.
def pluck(lst, key):
# Use a list comprehension to iterate through the dictionaries 'x' in the list 'lst'.
# For each dictionary 'x', get the value associated with the 'key' using the 'get' method.
# Return a list of these values.
return [x.get(key) for x in lst]
# Create a list of dictionaries 'simpsons' with 'name' and 'age' keys.
simpsons = [
{ 'name': 'Areeba', 'age': 8 },
{ 'name': 'Zachariah', 'age': 36 },
{ 'name': 'Caspar', 'age': 34 },
{ 'name': 'Presley', 'age': 10 }
]
# Call the 'pluck' function with the list 'simpsons' and the key 'age', and print the result.
print(pluck(simpsons, 'age'))
Sample Output:
[8, 36, 34, 10]
Flowchart:
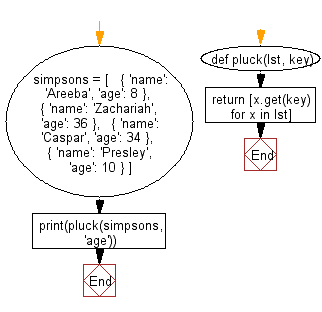
For more Practice: Solve these Related Problems:
- Write a Python program to extract values from a list of dictionaries for a specified key, skipping dictionaries where the key is missing.
- Write a Python program to convert a list of dictionaries into a list of values based on a nested key path.
- Write a Python program to extract and sort values corresponding to a given key from a list of dictionaries.
- Write a Python program to extract numeric values by key from a list of dictionaries and calculate their total sum.
Python Code Editor:
Previous: Write a Python program to find the items that are parity outliers in a given list.
Next: Write a Python program to calculate the average of a given list, after mapping each element to a value using the provided function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics