Python: Convert a given number (integer) to a list of digits
Convert Number to Digits List
Write a Python program to convert a given number (integer) to a list of digits.
Use map() combined with int on the string representation of n and return a list from the result.
Sample Solution:
Python Code:
# Define a function 'digitize' that takes an integer 'n' as input.
def digitize(n):
# Convert the integer 'n' to a string, map each character to an integer, and create a list of those integers.
return list(map(int, str(n)))
# Call the 'digitize' function with example integers and print the results.
print(digitize(123))
print(digitize(1347823))
Sample Output:
[1, 2, 3] [1, 3, 4, 7, 8, 2, 3]
Flowchart:
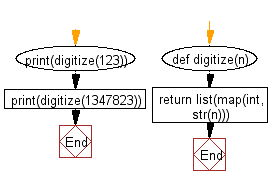
Python Code Editor:
Previous: Write a Python program to chunk a given list into n smaller lists.
Next: Write a Python program to find the index of the last element in the given list that satisfies the provided testing function.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics