Python: Create a list with the unique values filtered out
Unique Values in List
Write a Python program to create a list with unique values filtered out.
- Use collections.Counter to get the count of each value in the list.
- Use a list comprehension to create a list containing only the non-unique values.
Sample Solution:
Python Code:
# Import the 'Counter' class from the 'collections' module.
from collections import Counter
# Define a function called 'filter_unique' that takes a list 'lst' as an argument.
def filter_unique(lst):
# Create a list of items and their corresponding counts using the 'Counter' class.
# Filter this list to include only items with a count greater than 1.
return [item for item, count in Counter(lst).items() if count > 1]
# Example: Filter out unique elements from a list.
print(filter_unique([1, 2, 2, 3, 4, 4, 5]))
Sample Output:
[2, 4]
Flowchart:
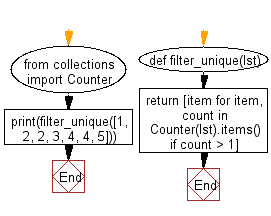
For more Practice: Solve these Related Problems:
- Write a Python program to extract only the unique values from a list, preserving the order of their first occurrence.
- Write a Python program to identify unique values in a list and then sort them in ascending order.
- Write a Python program to remove duplicate values from a list while maintaining the original order.
- Write a Python program to create a list of unique values from a nested list of numbers.
Go to:
Previous: Write a Python program to create a list with the non-unique values filtered out.
Next: Write a Python program to retrieve the value of the nested key indicated by the given selector list from a dictionary or list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.