Python: Builds a list, using an iterator function and an initial seed value
Build List Using Iterator
Write a Python program to build a list, using an iterator function and an initial seed value.
- The iterator function accepts one argument (seed) and must always return a list with two elements ([value, nextSeed]) or False to terminate.
- Use a generator function, fn_generator, that uses a while loop to call the iterator function and yield the value until it returns False.
- Use a list comprehension to return the list that is produced by the generator, using the iterator function.
Sample Solution:
Python Code:
# Define a function called 'unfold' that generates a list by repeatedly applying a function to a seed value.
def unfold(fn, seed):
# Define a generator function called 'fn_generator' that will yield values produced by the unfolding process.
def fn_generator(val):
while True:
# Apply the function 'fn' to the second element of 'val' (val[1]).
val = fn(val[1])
# If the result is False, stop the unfolding process.
if val == False:
break
# Yield the first element of 'val' (val[0]).
yield val[0]
# Create a list by collecting values from the generator using a list comprehension.
return [i for i in fn_generator([None, seed])]
# Define a lambda function 'f' that produces new values based on the input value.
f = lambda n: False if n > 40 else [-n, n + 10]
# Example usage: Use the 'unfold' function to generate a list of values based on the lambda function 'f' and an initial seed of 10.
print(unfold(f, 10))
Sample Output:
[-10, -20, -30, -40]
Flowchart:
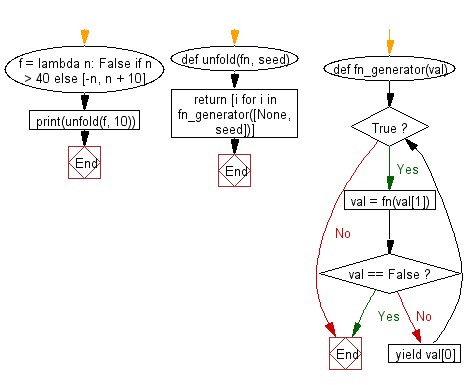
For more Practice: Solve these Related Problems:
- Write a Python program to build a list of powers of 2 using an iterator function starting from a given seed value.
- Write a Python program to generate the Fibonacci sequence as a list using an iterator function.
- Write a Python program to build a list where each element is obtained by multiplying the previous element by a fixed factor, using an iterator.
- Write a Python program to generate a list of successive factorial values using an iterator function and an initial seed value.
Go to:
Previous: Write a Python program to sort one list based on another list containing the desired indexes.
Next: Write a Python program to map the values of a list to a dictionary using a function, where the key-value pairs consist of the original value as the key and the result of the function as the value.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.