Python: Remove an element from a given list
Remove List Element by Index
Write a Python program to remove an element from a given list.
Sample Solution-1:
Python Code:
# Create a list 'student' containing mixed data types (strings and integers).
student = ['Ricky Rivera', 98, 'Math', 90, 'Science']
# Print a message indicating the original list.
print("Original list:")
# Print the original list 'student'.
print(student)
# Print a message indicating the purpose of the following lines of code.
print("\nAfter deleting an element, using the index of the element:")
# Use the 'del' statement to remove the element at index 0 from the 'student' list.
del(student[0])
# Print the updated 'student' list after deleting the element.
print(student)
Sample Output:
Original list: ['Ricky Rivera', 98, 'Math', 90, 'Science'] After deleting an element:, using index of the element: [98, 'Math', 90, 'Science']
Flowchart:
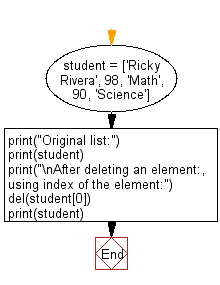
Sample Solution-2:
Python Code:
# Create a list 'student' containing mixed data types (strings and integers).
student = ['Ricky Rivera', 98, 'Math', 90, 'Science']
# Print a message indicating the original list.
print("Original list:")
# Print the original list 'student'.
print(student)
# Print a message indicating the purpose of the following lines of code.
print("\nAfter deleting an element, using the 'remove' function:")
# Use the 'remove' function to remove the first occurrence of the string 'Math' from the 'student' list.
student.remove('Math')
# Print the updated 'student' list after removing the element 'Math'.
print(student)
Sample Output:
Original list: ['Ricky Rivera', 98, 'Math', 90, 'Science'] After deleting an element:, using remove function: ['Ricky Rivera', 98, 90, 'Science']
Flowchart:
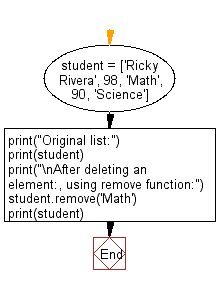
Sample Solution-3:
Python Code:
# Create a list 'student' containing mixed data types (strings and integers).
student = ['Ricky Rivera', 98, 'Math', 90, 'Science']
# Print a message indicating the original list.
print("Original list:")
# Print the original list 'student'.
print(student)
# Print a message indicating the purpose of the following lines of code.
print("\nAfter deleting an element, using the 'pop' function:")
# Use the 'pop' function to remove the last element from the 'student' list, and assign the removed value to 'z'.
z = student.pop()
# Print the updated 'student' list after removing the last element.
print(student)
# Print the removed value stored in 'z'.
print("Removed value is:", z)
Sample Output:
Original list: ['Ricky Rivera', 98, 'Math', 90, 'Science'] After deleting an element:, using pop function: ['Ricky Rivera', 98, 'Math', 90] Removed value is: Science
Flowchart:
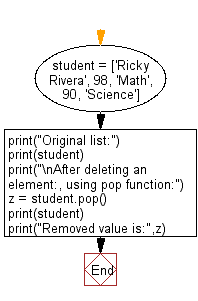
Python Code Editor:
Previous: Write a Python program to compute the sum of non-zero groups (separated by zeros) of a given list of numbers.
Next: Write a Python program to remove all the values except integer values from a given array of mixed values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics