Python: Remove additional spaces in a given list
Remove Extra Spaces from List
Write a Python program to remove additional spaces from a given list.
Sample Solution:
Python Code:
# Define a function 'test' that removes additional spaces from the elements of a given list.
def test(lst):
# Create an empty list 'result' to store the modified elements.
result = []
# Iterate through the elements of the input list 'lst'.
for i in lst:
# Remove extra spaces in the current element and store it in 'j'.
j = i.replace(' ', '')
# Append the modified element 'j' to the 'result' list.
result.append(j)
return result
# Define a list 'text' containing strings with extra spaces.
text = ['abc ', ' ', ' ', 'sdfds ', ' ', ' ', 'sdfds ', 'huy']
# Print a message indicating the original list.
print("\nOriginal list:")
# Print the original list 'text'.
print(text)
# Print a message indicating the purpose of the following lines of code.
print("Remove additional spaces from the said list:")
# Call the 'test' function to remove extra spaces from the elements of 'text' and print the result.
print(test(text))
Sample Output:
Original list: ['abc ', ' ', ' ', 'sdfds ', ' ', ' ', 'sdfds ', 'huy'] Remove additional spaces from the said list: ['abc', '', '', 'sdfds', '', '', 'sdfds', 'huy']
Flowchart:
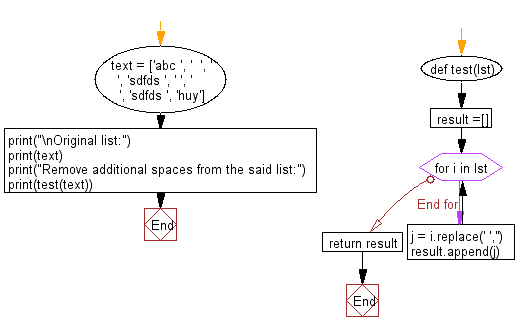
For more Practice: Solve these Related Problems:
- Write a Python program to trim whitespace from the beginning and end of each string in a list.
- Write a Python program to remove only the leading spaces from each element in a list of strings.
- Write a Python program to remove extra spaces from a list and join the non-empty strings into a single sentence.
- Write a Python program to replace multiple consecutive spaces within each string in a list with a single space.
Python Code Editor:
Previous: Write a Python program to find the indices of elements of a given list, greater than a specified value.
Next: Write a Python program to find the common tuples between two given lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.