Python: Find the dimension of a given matrix
Matrix Dimensions
Write a Python program to find the dimension of a given matrix.
Visual Presentation:
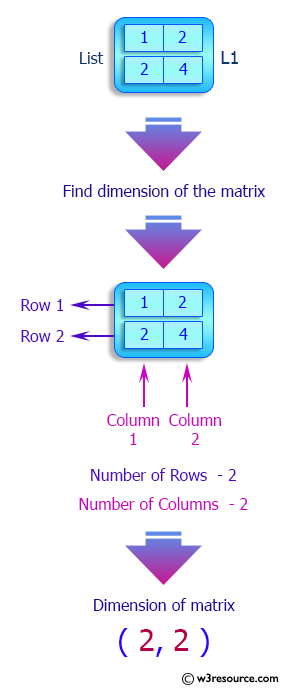
Sample Solution:
Python Code:
# Define a function called 'matrix_dimensions' that calculates the number of rows and columns in a matrix (list of lists).
def matrix_dimensions(test_list):
# Calculate the number of rows by finding the length of the input list.
row = len(test_list)
# Calculate the number of columns by finding the length of the first sub-list (assuming all sub-lists have the same length).
column = len(test_list[0])
return row, column # Return the number of rows and columns as a tuple.
# Create a matrix 'lst' represented as a list of lists.
lst = [[1, 2], [2, 4]]
# Print a message indicating the original list (matrix).
print("\nOriginal list:")
# Print the original matrix 'lst'.
print(lst)
# Print a message indicating the dimensions of the matrix.
print("Dimension of the said matrix:")
# Call the 'matrix_dimensions' function with 'lst' and print the result (number of rows and columns).
print(matrix_dimensions(lst))
# Create another matrix 'lst' with a different number of rows and columns.
lst = [[0, 1, 2], [2, 4, 5]]
# Print a message indicating the original list (matrix).
print("\nOriginal list:")
# Print the original matrix 'lst'.
print(lst)
# Print a message indicating the dimensions of the matrix.
print("Dimension of the said matrix:")
# Call the 'matrix_dimensions' function with 'lst' and print the result (number of rows and columns).
print(matrix_dimensions(lst))
# Create another matrix 'lst' with a different number of rows and columns.
lst = [[0, 1, 2], [2, 4, 5], [2, 3, 4]]
# Print a message indicating the original list (matrix).
print("\nOriginal list:")
# Print the original matrix 'lst'.
print(lst)
# Print a message indicating the dimensions of the matrix.
print("Dimension of the said matrix:")
# Call the 'matrix_dimensions' function with 'lst' and print the result (number of rows and columns).
print(matrix_dimensions(lst))
Sample Output:
Original list: [[1, 2], [2, 4]] Dimension of the said matrix: (2, 2) Original list: [[0, 1, 2], [2, 4, 5]] Dimension of the said matrix: (2, 3) Original list: [[0, 1, 2], [2, 4, 5], [2, 3, 4]] Dimension of the said matrix: (3, 3)
Flowchart:
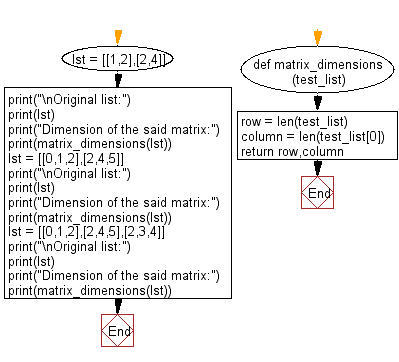
For more Practice: Solve these Related Problems:
- Write a Python program to determine the dimensions of a matrix and check if it is a square matrix.
- Write a Python program to compute the dimensions of multiple matrices and compare them for uniformity.
- Write a Python program to find the dimensions of a matrix and then compute its transpose.
- Write a Python program to determine the dimensions of an irregular matrix, treating missing elements as None.
Go to:
Previous: Write a Python program to remove all strings from a given list of tuples.
Next: Write a Python program to sum two or more lists, the lengths of the lists may be different.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.