Python: Remove all strings from a given list of tuples
Remove Strings from List of Tuples
Write a Python program to remove all strings from a given list of tuples.
Sample Solution:
Python Code:
# Define a function called 'test' that removes strings from a list of tuples.
def test(list1):
# Create a list comprehension that processes each tuple in the input list.
# Within each tuple, create a new tuple that contains values that are not strings.
result = [tuple(v for v in i if not isinstance(v, str)) for i in list1]
return list(result) # Return the resulting list of tuples.
# Create a list of tuples 'marks', where each tuple contains numbers and strings.
marks = [(100, 'Math'), (80, 'Math'), (90, 'Math'), (88, 'Science', 89), (90, 'Science', 92)]
# Print a message indicating the original list of tuples.
print("\nOriginal list:")
# Print the original list 'marks'.
print(marks)
# Print a message indicating the result after removing strings from the list of tuples.
print("\nRemove all strings from the said list of tuples:")
# Call the 'test' function with 'marks' and print the result.
print(test(marks))
Sample Output:
Original list: [(100, 'Math'), (80, 'Math'), (90, 'Math'), (88, 'Science', 89), (90, 'Science', 92)] Remove all strings from the said list of tuples: [(100,), (80,), (90,), (88, 89), (90, 92)]
Flowchart:
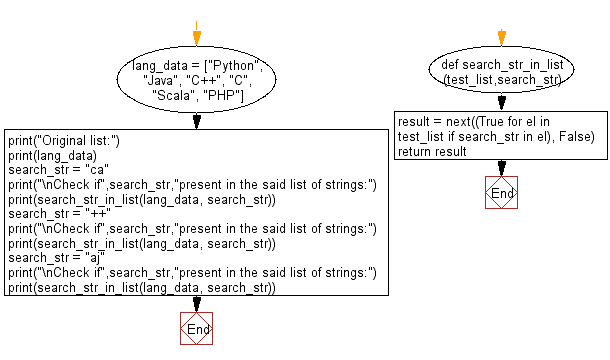
For more Practice: Solve these Related Problems:
- Write a Python program to remove all string elements from a list of tuples and replace them with their character counts.
- Write a Python program to remove strings from a list of tuples only if they start with a vowel.
- Write a Python program to remove all strings from a list of tuples and then sum the remaining numeric values for each tuple.
- Write a Python program to remove strings from a list of tuples and output a new list containing only the numeric values.
Python Code Editor:
Previous: Write a Python program to find the maximum and minimum value of the three given lists.
Next: Write a Python program to find the dimension of a given matrix.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics