Python: Swap two sublists in a given list
Swap Sublists in List
Write a Python program to swap two sublists in a given list.
Sample Solution:
Python Code:
# Create a list 'nums' containing integer values from 0 to 15.
nums = [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15]
# Print a message indicating the original list.
print("Original list:")
# Print the original list 'nums'.
print(nums)
# Swap two sublists in 'nums'. The sublist from index 6 to 10 is swapped with the sublist from index 1 to 3.
nums[6:10], nums[1:3] = nums[1:3], nums[6:10]
# Print a message indicating that two sublists have been swapped.
print("\nSwap two sublists of the said list:")
# Print the modified 'nums' list with the swapped sublists.
print(nums)
# Swap two sublists in 'nums'. The sublist from index 1 to 3 is swapped with the sublist from index 4 to 6.
nums[1:3], nums[4:6] = nums[4:6], nums[1:3]
# Print a message indicating that two sublists have been swapped again.
print("\nSwap two sublists of the said list:")
# Print the modified 'nums' list with the sublists swapped back to their original positions.
print(nums)
Sample Output:
Original list: [0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15] Swap two sublists of the said list: [0, 6, 7, 8, 9, 3, 4, 5, 1, 2, 10, 11, 12, 13, 14, 15] Swap two sublists of the said list: [0, 9, 3, 8, 6, 7, 4, 5, 1, 2, 10, 11, 12, 13, 14, 15]
Flowchart:
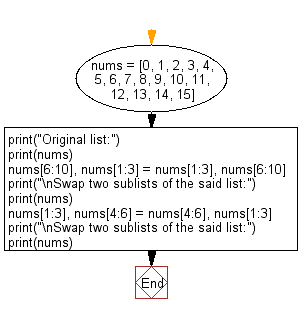
For more Practice: Solve these Related Problems:
- Write a Python program to swap two non-overlapping sublists in a list given their index ranges.
- Write a Python program to swap the first half and the second half of a list.
- Write a Python program to swap the sublist before a given index with the sublist after that index.
- Write a Python program to swap two sublists only if they are of equal length.
Go to:
Previous: Write a Python program to convert a given decimal number to binary list.
Next: Write a Python program to convert a given list of tuples to a list of strings.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.