Python: Convert a given decimal number to binary list
Convert Decimal to Binary List
Write a Python program to convert a given decimal number to a binary list.
Visual Presentation:
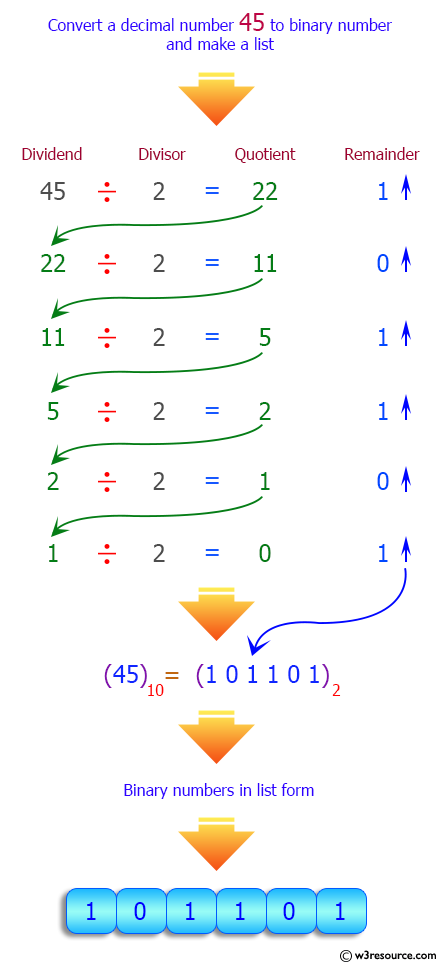
Sample Solution:
Python Code:
# Define a function called 'decimal_to_binary_list' that converts a decimal number to a binary list.
def decimal_to_binary_list(n):
result = [int(x) for x in list('{0:0b}'.format(n))]
# Convert the decimal number 'n' to a binary string, convert each digit to an integer, and store in a list.
return result
# Set the value of 'n' to 8.
n = 8
# Print a message indicating the original number.
print("Original Number:", n)
# Call the 'decimal_to_binary_list' function with 'n' and print the result.
print("Decimal number (", n, ") to binary list:")
# Print the binary representation of the number 8 as a list.
print(decimal_to_binary_list(n))
# Set the value of 'n' to 45.
n = 45
# Print a message indicating the original number.
print("\nOriginal Number:", n)
# Call the 'decimal_to_binary_list' function with 'n' and print the result.
print("Decimal number (", n, ") to binary list:")
# Print the binary representation of the number 45 as a list.
print(decimal_to_binary_list(n))
# Set the value of 'n' to 100.
n = 100
# Print a message indicating the original number.
print("\nOriginal Number:", n)
# Call the 'decimal_to_binary_list' function with 'n' and print the result.
print("Decimal number (", n, ") to binary list:")
# Print the binary representation of the number 100 as a list.
print(decimal_to_binary_list(n))
Sample Output:
Original Number: 8 Decimal number ( 8 ) to binary list: [1, 0, 0, 0] Original Number: 45 Decimal number ( 45 ) to binary list: [1, 0, 1, 1, 0, 1] Original Number: 100 Decimal number ( 100 ) to binary list: [1, 1, 0, 0, 1, 0, 0]
Flowchart:
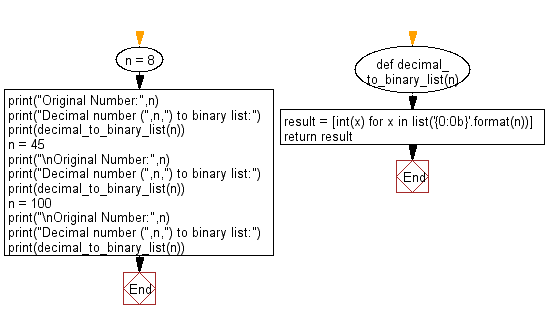
For more Practice: Solve these Related Problems:
- Write a Python program to convert a given decimal number to a binary list and then invert all binary digits.
- Write a Python program to convert a decimal number to a binary list and pad it to a specified length with leading zeros.
- Write a Python program to convert a decimal number to a binary list and count the number of 1's in the binary representation.
- Write a Python program to convert a decimal number to a binary list and then reverse the binary list.
Go to:
Previous: Write a Python program to form Bigrams of words in a given list of strings.
Next: Write a Python program to swap two sublists in a given list.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.