Python: Add two given lists of different lengths, start from right
Add Lists from Right
Write a Python program to add two given lists of different lengths, starting on the right.
Sample Solution:
Python Code:
def elementswise_right_join(l1, l2):
f_len = len(l1)-(len(l2) - 1)
for i in range(len(l1), 0, -1):
if i-f_len < 0:
break
else:
l1[i-1] = l1[i-1] + l2[i-f_len]
return l1
nums1 = [2, 4, 7, 0, 5, 8]
nums2 = [3, 3, -1, 7]
print("\nOriginal lists:")
print(nums1)
print(nums2)
print("\nAdd said two lists from left:")
print(elementswise_right_join(nums1, nums2))
nums3 = [1, 2, 3, 4, 5, 6]
nums4 = [2, 4, -3]
print("\nOriginal lists:")
print(nums3)
print(nums4)
print("\nAdd said two lists from left:")
print(elementswise_right_join(nums3, nums4))
Sample Output:
Original lists: [2, 4, 7, 0, 5, 8] [3, 3, -1, 7] Add said two lists from left: [2, 4, 10, 3, 4, 15] Original lists: [1, 2, 3, 4, 5, 6] [2, 4, -3] Add said two lists from left: [1, 2, 3, 6, 9, 3]
Flowchart:
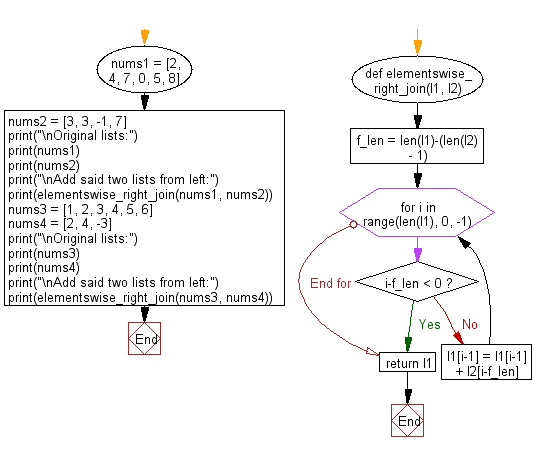
Python Code Editor:
Previous: Write a Python program to add two given lists of different lengths, start from left.
Next: Write a Python program to interleave multiple given lists of different lengths.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics