Python: Interleave two given list into another list randomly
Randomly Interleave Two Lists
Write a Python program to combine two lists into another list randomly.
Sample Solution:
Python Code:
# Import the 'random' module to generate random values
import random
# Define a function 'randomly_interleave' that interleaves two lists randomly
def randomly_interleave(nums1, nums2):
# Create a new list by sampling elements from 'nums1' and 'nums2'
# The elements from 'nums1' and 'nums2' are interleaved randomly
result = [x.pop(0) for x in random.sample([nums1] * len(nums1) + [nums2] * len(nums2), len(nums1) + len(nums2))]
return result
# Create two lists 'nums1' and 'nums2'
nums1 = [1, 2, 7, 8, 3, 7]
nums2 = [4, 3, 8, 9, 4, 3, 8, 9]
# Print a message indicating the original lists
print("Original lists:")
# Print the contents of 'nums1' and 'nums2'
print(nums1)
print(nums2)
# Print a message indicating the operation to interleave the two lists
print("\nInterleave two given lists into another list randomly:")
# Call the 'randomly_interleave' function with 'nums1' and 'nums2', then print the result
print(randomly_interleave(nums1, nums2))
Sample Output:
Original lists: [1, 2, 7, 8, 3, 7] [4, 3, 8, 9, 4, 3, 8, 9] Interleave two given list into another list randomly: [4, 1, 2, 3, 8, 9, 4, 3, 7, 8, 9, 8, 3, 7]
Flowchart:
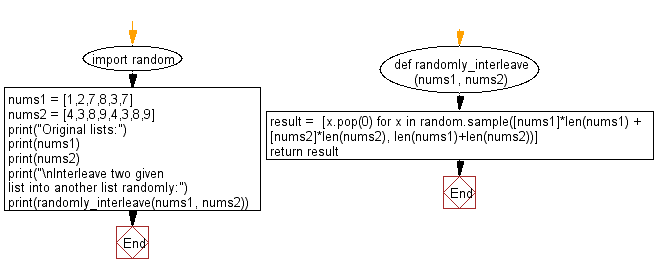
For more Practice: Solve these Related Problems:
- Write a Python program to interleave two lists in random order while ensuring no duplicates are adjacent.
- Write a Python program to randomly interleave two lists while keeping elements in pairs.
- Write a Python program to interleave two lists randomly but ensure the first list contributes more elements.
- Write a Python program to interleave two lists randomly while ensuring the resulting list maintains alternating elements.
Python Code Editor:
Previous: Write a Python program to compute the sum of digits of each number of a given list.
Next: Write a Python program to remove specific words from a given list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics