Python: Sort a given mixed list of integers and strings
Sort Mixed List (Numbers Before Strings)
Write a Python program to sort a given mixed list of integers and strings. Numbers must be sorted before strings.
Sample Solution:
Python Code:
# Define a function 'sort_mixed_list' that sorts a mixed list of integers and strings
def sort_mixed_list(mixed_list):
# Extract and sort the integer part of the list
int_part = sorted([i for i in mixed_list if type(i) is int])
# Extract and sort the string part of the list
str_part = sorted([i for i in mixed_list if type(i) is str])
# Combine the sorted integer and string parts and return the result
return int_part + str_part
# Create a mixed list of integers and strings 'mixed_list'
mixed_list = [19, 'red', 12, 'green', 'blue', 10, 'white', 'green', 1]
# Print a message indicating the original mixed list
print("Original list:")
# Print the contents of 'mixed_list'
print(mixed_list)
# Sort the mixed list of integers and strings using the 'sort_mixed_list' function
print("\nSort the said mixed list of integers and strings:")
# Call the 'sort_mixed_list' function with 'mixed_list', then print the result
print(sort_mixed_list(mixed_list))
Sample Output:
Original list: [19, 'red', 12, 'green', 'blue', 10, 'white', 'green', 1] Sort the said mixed list of integers and strings: [1, 10, 12, 19, 'blue', 'green', 'green', 'red', 'white']
Flowchart:
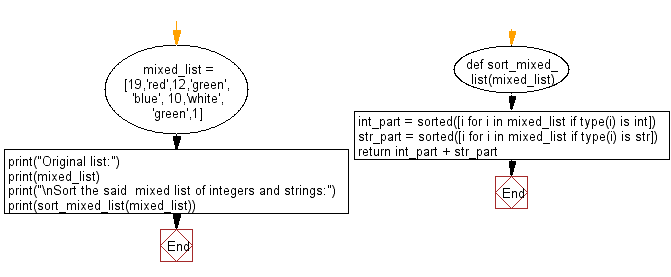
Python Code Editor:
Previous: Write a Python program to find a first even and odd number in a given list of numbers.
Next: Write a Python program to sort a given list of strings(numbers) numerically.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics