Python: Remove duplicate words from a given list of strings
Remove Duplicate Words from List
Write a Python program to remove duplicate words from a given list of strings.
Sample Solution:
Python Code:
# Define a function 'unique_list' that removes duplicates from a list
def unique_list(l):
# Create an empty list 'temp' to store unique elements
temp = []
# Iterate through the elements of the input list 'l'
for x in l:
# Check if the element 'x' is not in the 'temp' list (i.e., it's unique)
if x not in temp:
# Append the unique element 'x' to the 'temp' list
temp.append(x)
# Return the list of unique elements
return temp
# Create a list of strings 'text_str' with duplicate words
text_str = ["Python", "Exercises", "Practice", "Solution", "Exercises"]
# Print a message indicating the original list of strings
print("Original String:")
# Print the contents of 'text_str'
print(text_str)
# Remove duplicate words from the list of strings using the 'unique_list' function
print("\nAfter removing duplicate words from the said list of strings:")
# Call the 'unique_list' function with 'text_str', then print the result
print(unique_list(text_str))
Sample Output:
Original String: ['Python', 'Exercises', 'Practice', 'Solution', 'Exercises'] After removing duplicate words from the said list of strings: ['Python', 'Exercises', 'Practice', 'Solution']
Flowchart:
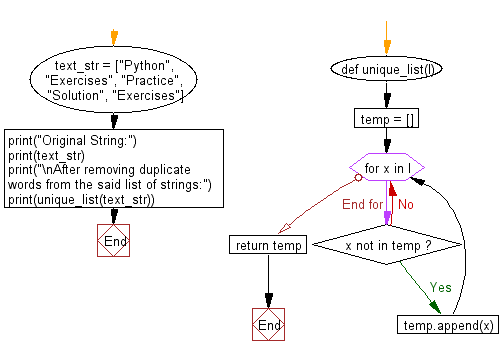
Python Code Editor:
Previous: Write a Python program to iterate over all pairs of consecutive items in a given list.
Next: Write a Python program to find a first even and odd number in a given list of numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics