Python: Count the same pair in three given lists
Count Same Pairs in Three Lists
Write a Python program to count the same pair in three given lists.
Sample Solution:
Python Code:
Sample Output:
Original lists: [1, 2, 3, 4, 5, 6, 7, 8] [2, 2, 3, 1, 2, 6, 7, 9] [2, 1, 3, 1, 2, 6, 7, 9] Number of same pairs in the said three given lists: 3
Flowchart:
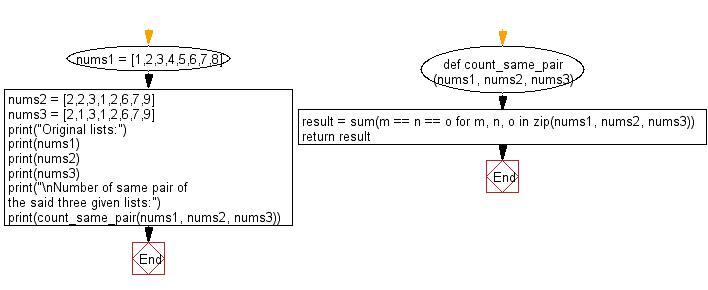
Python Code Editor:
Previous: Write a Python program to reverse each list in a given list of lists.
Next: Write a Python program to count the frequency of consecutive duplicate elements in a given list of numbers.
What is the difficulty level of this exercise?
Based on 2 votes, average difficulty level of this exercise is Hard
.
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics