Python: Find the difference between consecutive numbers in a given list
Python List: Exercise - 104 with Solution
Difference Between Consecutive Numbers
Write a Python program to find the difference between consecutive numbers in a given list.
Visual Presentation:
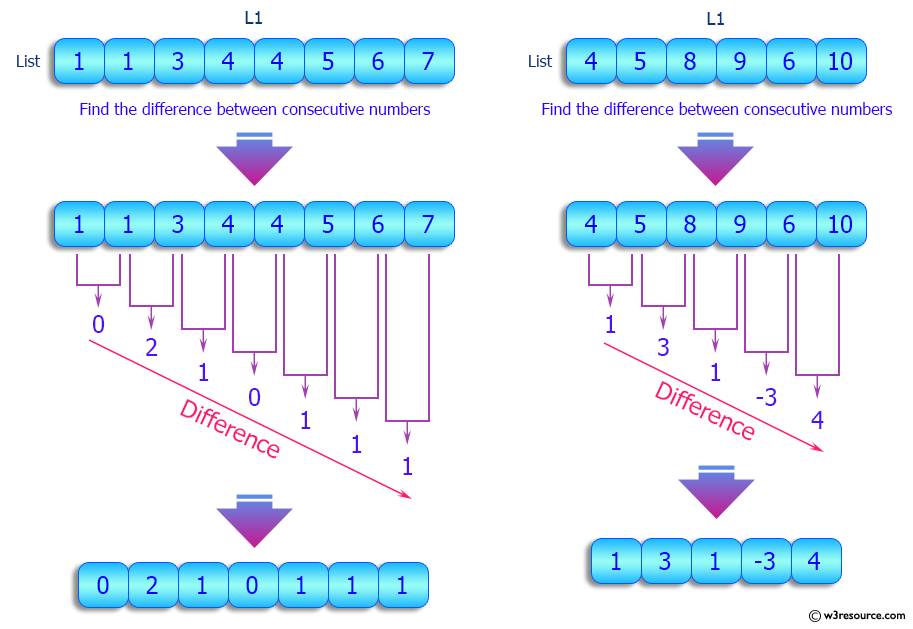
Sample Solution:
Python Code:
# Define a function 'diff_consecutive_nums' that calculates the differences between consecutive numbers in a list
def diff_consecutive_nums(nums):
# Use a list comprehension and the 'zip' function to calculate differences between consecutive numbers
result = [b - a for a, b in zip(nums[:-1], nums[1:])]
return result
# Create a list 'nums1' containing numbers
nums1 = [1, 1, 3, 4, 4, 5, 6, 7]
# Print a message indicating the original list
print("Original list:")
# Print the contents of 'nums1'
print(nums1)
# Print a message indicating that the differences between consecutive numbers will be determined
print("Difference between consecutive numbers of the said list:")
# Call the 'diff_consecutive_nums' function with 'nums1' and print the result
print(diff_consecutive_nums(nums1))
# Create a list 'nums2' containing numbers
nums2 = [4, 5, 8, 9, 6, 10]
# Print a message indicating the original list
print("\nOriginal list:")
# Print the contents of 'nums2'
print(nums2)
# Print a message indicating that the differences between consecutive numbers will be determined
print("Difference between consecutive numbers of the said list:")
# Call the 'diff_consecutive_nums' function with 'nums2' and print the result
print(diff_consecutive_nums(nums2))
Sample Output:
Original list: [1, 1, 3, 4, 4, 5, 6, 7] Difference between consecutive numbers of the said list: [0, 2, 1, 0, 1, 1, 1] Original list: [4, 5, 8, 9, 6, 10] Difference between consecutive numbers of the said list: [1, 3, 1, -3, 4]
Flowchart:
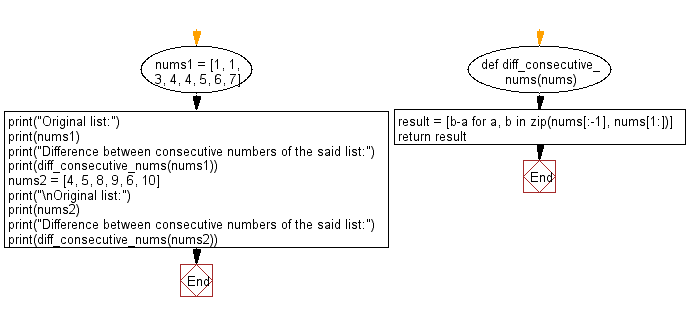
Python Code Editor:
Previous: Write a Python program to extract specified number of elements from a given list, which follows each other continuously.
Next: Write a Python program to compute average of two given lists.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/list/python-data-type-list-exercise-104.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics