Python: Sort a given list of elements in ascending order using Heap queue algorithm
6. Heapsort Ascending Order
Write a Python program to sort a given list of elements in ascending order using the heap queue algorithm.
Sample Solution:
Python Code:
import heapq as hq
nums_list = [18, 14, 10, 9, 8, 7, 9, 3, 2, 4, 1]
print("Original list:")
print(nums_list)
hq.heapify(nums_list)
s_result = [hq.heappop(nums_list) for i in range(len(nums_list))]
print("\nSorted list:")
print(s_result)
Sample Output:
Original list: [18, 14, 10, 9, 8, 7, 9, 3, 2, 4, 1] Sorted list: [1, 2, 3, 4, 7, 8, 9, 9, 10, 14, 18]
Flowchart:
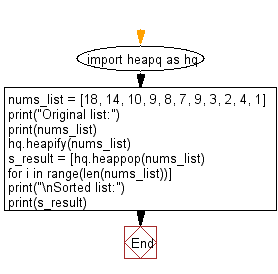
For more Practice: Solve these Related Problems:
- Write a Python program to sort a list in ascending order by first converting it into a heap and then continuously popping the smallest element.
- Write a Python script to implement heapsort using heapq.heapify and heappop, and then verify that the resulting list is sorted.
- Write a Python function that sorts a list using the heap queue algorithm and prints both the original and the sorted lists.
- Write a Python program to demonstrate heapsort on a list of mixed integers and output the sorted array along with the number of heappop operations.
Go to:
Previous: Write a Python program to delete the smallest element from the given Heap and then inserts a new item.
Next: Write a Python program to find the kth largest element in an unsorted array using Heap queue algorithm.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.