Python: Create a FIFO queue
28. FIFO Queue Creation
Write a Python program to create a FIFO queue.
Sample Solution:
Python Code:
import queue
q = queue.Queue()
#insert items at the end of the queue
for x in range(4):
q.put(str(x))
#remove items from the head of the queue
while not q.empty():
print(q.get(), end=" ")
print("\n")
Sample Output:
0 1 2 3
Flowchart:
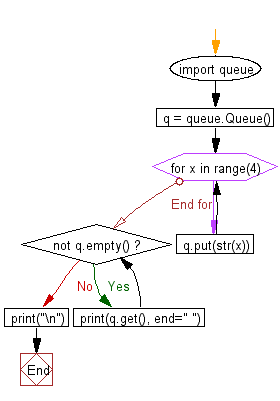
For more Practice: Solve these Related Problems:
- Write a Python program to create a FIFO queue using collections.deque, enqueue a series of elements, and then display them in order.
- Write a Python script to simulate a FIFO queue by adding items and then dequeuing them one by one, printing each item as it is removed.
- Write a Python program to implement a FIFO queue, display its contents before and after dequeuing, and then print the final queue size.
- Write a Python function to create a FIFO queue, add several numbers, and then return the queue as a list in the order they were enqueued.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to find whether a queue is empty or not.
Next: Write a Python program to create a LIFO queue.What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.