Python: Push three items into the heap and print the items from the heap
Python heap queue algorithm: Exercise-21 with Solution
Write a Python program to push three items into the heap and print the items from the heap.
Sample Solution:
Python Code:
import heapq
heap = []
heapq.heappush(heap, ('V', 1))
heapq.heappush(heap, ('V', 2))
heapq.heappush(heap, ('V', 3))
for a in heap:
print(a)
Sample Output:
('V', 1) ('V', 2) ('V', 3)
Flowchart:
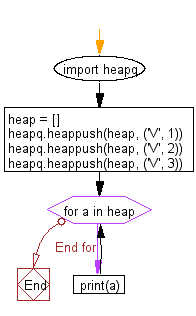
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to combine two given sorted lists using heapq module.
Next: Write a Python program to push three items into a heap and return the smallest item from the heap. Also Pop and return the smallest item from the heap.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/heap-queue-algorithm/python-heapq-exercise-21.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics