Python function to filter strings with a specific substring
9. Substring Excluder
Write a Python function that filters out elements from a list of strings containing a specific substring using the filter function.
Sample Solution:
Python Code:
def filter_strings_with_substring(strings, substring):
"""
Filters out elements from a list of strings containing a specific substring.
Args:
strings (list): A list of strings.
substring (str): The substring to search for in the strings.
Returns:
list: A new list containing only the strings that contain the substring.
"""
# Define the filtering function
def contains_substring(string):
return substring in string
# Use the filter function to filter out strings with the substring
filtered_strings = list(filter(contains_substring, strings))
return filtered_strings
# Example usage:
strings = ["Red", "Green", "Orange", "White", "Black", "Pink", "Yellow"]
print("List of words:")
print(strings)
substring = "l"
print("Substring:",substring)
print("Filter out strings with the substring:")
result = filter_strings_with_substring(strings, substring)
print(result)
Explanation:
In the exercise above -
- First, the "filter_strings_with_substring()" function takes two arguments: strings (a list of strings) and substring (the substring to search for).
- Inside the function, we define a nested function "contains_substring()" that checks if a string contains the specified substring using the in operator.
- Use the filter function to filter out strings from the strings list by applying the "contains_substring()" function as the filtering condition.
- Finally, the filtered strings containing the specified substring are converted to a list and returned as the result.
Sample Output:
List of words: ['Red', 'Green', 'Orange', 'White', 'Black', 'Pink', 'Yellow'] Substring: l Filter out strings with the substring: ['Black', 'Yellow']
Flowchart:
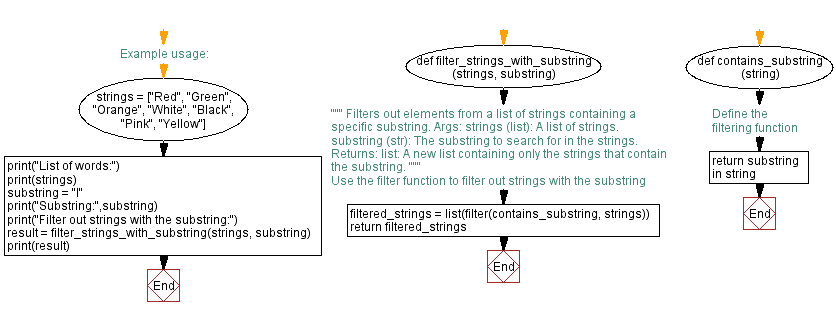
For more Practice: Solve these Related Problems:
- Write a Python program to filter out strings containing a specific substring and then return the remaining strings sorted by their lengths.
- Write a Python function that uses the filter function to remove strings containing a given substring and then converts the remaining strings to lowercase.
- Write a Python program to filter a list of strings to exclude those with a specific substring, and then concatenate the remaining strings with a space separator.
- Write a Python function that filters a list of strings, retaining only those that do not contain a given substring, and then counts the number of retained strings.
Go to:
Previous: Python program to extract words with more than five letters.
Next: Python program to filter future dates.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.