Python program to filter students by high grades
Python Filter: Exercise-6 with Solution
Write a Python program that creates a list of dictionaries containing student information (name, age, grade) and uses the filter function to extract students with a grade greater than or equal to 95.
Sample Solution:
Python Code:
# Define a list of dictionaries containing student information
students = [
{"name": "Denis Helio", "age": 17, "grade": 97},
{"name": "Hania Mehtap", "age": 16, "grade": 92},
{"name": "Kelan Stasys", "age": 17, "grade": 90},
{"name": "Velvet Mirko", "age": 16, "grade": 94},
{"name": "Delores Aeneas", "age": 17, "grade": 100},
]
print("Student information:")
print(students)
# Define a function to check if a student's grade is greater than or equal to 95
def has_high_grade(student):
return student["grade"] >= 95
# Use the filter function to extract students with high grades
high_grade_students = list(filter(has_high_grade, students))
print("\nStudents with high grades:")
# Print the extracted students
print(high_grade_students)
Explanation:
In the exercise above -
- First, we define a list called students, where each element is a dictionary containing information about a student (name, age, and grade).
- Next, we define a function called "has_high_grade()" that checks if a student's grade is greater than or equal to 95.
- Finally, we use the filter function to filter the students from the students list based on the condition defined by the "has_high_grade()" function.
- The filtered students with high grades are converted to a list, and we print the extracted students.
Sample Output:
Student information: [{'name': 'Denis Helio', 'age': 17, 'grade': 97}, {'name': 'Hania Mehtap', 'age': 16, 'grade': 92}, {'name': 'Kelan Stasys', 'age': 17, 'grade': 90}, {'name': 'Velvet Mirko', 'age': 16, 'grade': 94}, {'name': 'Delores Aeneas', 'age': 17, 'grade': 100}] Students with high grades: [{'name': 'Denis Helio', 'age': 17, 'grade': 97}, {'name': 'Delores Aeneas', 'age': 17, 'grade': 100}]
Flowchart:
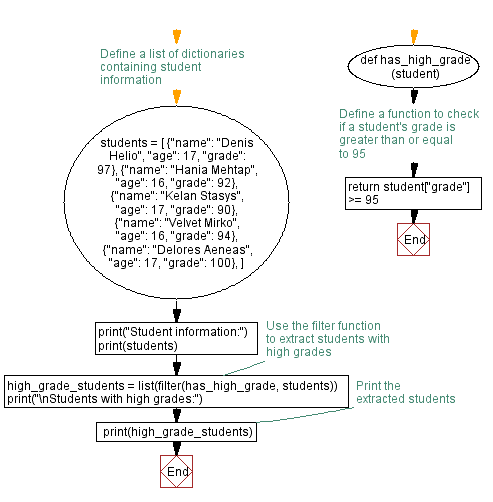
Python Code Editor:
Previous: Python function to filter out empty strings from a list.
Next: Python program to filter prime numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics