Python function to filter out empty strings from a list
Python Filter: Exercise-5 with Solution
Write a Python function that filters out all empty strings from a list of strings using the filter function.
Sample Solution:
Python Code:
def filter_non_empty_strings(strings):
"""
Filters out all empty strings from a list of strings.
Args:
strings (list): A list of strings.
Returns:
list: A new list containing only non-empty strings.
"""
# Define the filtering function
def is_non_empty_string(s):
return s.strip() != ""
# Use the filter function to filter out empty strings
non_empty_strings = list(filter(is_non_empty_string, strings))
return non_empty_strings
# Example usage:
strings = ["", "w3resource", "Filter", "", "Python", ""]
print("Original list of strings:",strings)
print("\nA new list containing only non-empty strings:")
result = filter_non_empty_strings(strings)
print(result)
Explanation:
In the exercise above -
- First, the "filter_non_empty_strings()" function takes a list of strings called strings as input.
- Inside the function, we define a nested function "is_non_empty_string()" that checks if a string is non-empty by using the strip() method to remove leading and trailing whitespace and then checking if it's an empty string.
- We use the filter() function to filter out empty strings from the strings list by applying the "is_non_empty_string()" function as the filtering condition.
- The filtered 'non_empty_strings' are converted to a list and returned as the result.
Sample Output:
Original list of strings: ['', 'w3resource', 'Filter', '', 'Python', ''] A new list containing only non-empty strings: ['w3resource', 'Filter', 'Python']
Flowchart:
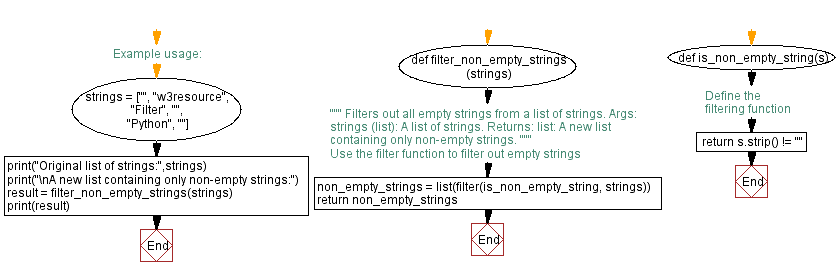
Python Code Editor:
Previous: Python program to extract names starting with vowels.
Next: Python program to filter students by high grades.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics