Python program to filter future dates
10. Future Date Filter
Write a Python program that implements a Python program that filters out dates (in the format "YYYY-MM-DD") that are in the future using the filter function.
Sample Solution:
Python Code:
from datetime import datetime
# Define a list of dates in "YYYY-MM-DD" format
date_strings = ["2023-07-11", "2022-02-22", "2024-05-11", "2025-12-31", "2021-01-01"]
print("List of dates:")
print(date_strings)
# Convert the date strings to datetime objects
dates = [datetime.strptime(date, "%Y-%m-%d") for date in date_strings]
# Get the current date
current_date = datetime.now()
print("Current date:",current_date)
# Define a function to check if a date is in the future
def is_date_in_future(date):
return date > current_date
# Use the filter function to extract dates in the past
dates_in_past = list(filter(is_date_in_future, dates))
# Convert the filtered dates back to strings
filtered_date_strings = [date.strftime("%Y-%m-%d") for date in dates_in_past]
print("\nCheck if a date is in the future!")
# Print the dates in the past
print(filtered_date_strings)
Explanation:
In the exercise above -
- First, we start with a list of date strings in the "YYYY-MM-DD" format.
- Convert date strings to datetime objects using datetime.strptime.
- We get the current date and time using datetime.now().
- Define a function "is_date_in_future()" that checks if a date is in the future by comparing it with the current date.
- Use the filter function to filter out dates in the future from the dates list by applying the "is_date_in_future()" function as the filtering condition.
- The filtered datetime objects are then converted back to date strings using strftime with the "YYYY-MM-DD" format.
- Finally, we print the dates in the future.
Sample Output:
List of dates: ['2023-07-11', '2022-02-22', '2024-05-11', '2025-12-31', '2021-01-01'] Current date: 2023-09-30 10:04:30.206035 Check if a date is in the future! ['2024-05-11', '2025-12-31']
Flowchart:
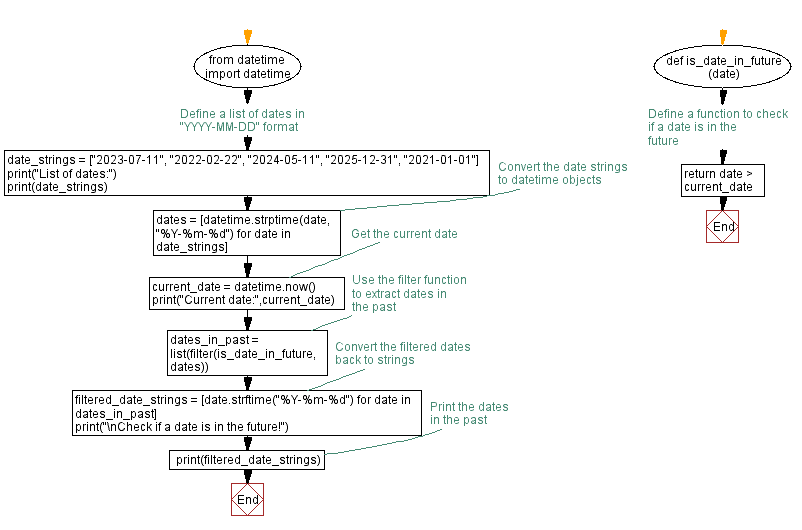
For more Practice: Solve these Related Problems:
- Write a Python program to filter out dates in the future from a list of 'YYYY-MM-DD' formatted strings and return only the past dates sorted in descending order.
- Write a Python function that uses the filter function to separate dates from the current year and those in the future.
- Write a Python program to filter a list of dates, excluding those that are after today, and then reformat the remaining dates to 'DD/MM/YYYY'.
- Write a Python program to filter out future dates from a list and then calculate the number of days each remaining date is from today.
Go to:
Previous: Python function to filter strings with a specific substring.
Next: Python program to filter cities by population.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.