Python File I/O: Read last n lines of a file
Write a Python program to read last n lines of a file.
Sample Solution:-
Python Code:
import sys
import os
def file_read_from_tail(fname,lines):
bufsize = 8192
fsize = os.stat(fname).st_size
iter = 0
with open(fname) as f:
if bufsize > fsize:
bufsize = fsize-1
data = []
while True:
iter +=1
f.seek(fsize-bufsize*iter)
data.extend(f.readlines())
if len(data) >= lines or f.tell() == 0:
print(''.join(data[-lines:]))
break
file_read_from_tail('test.txt',2)
Sample Output:
Append this text. Append this text.
Flowchart:
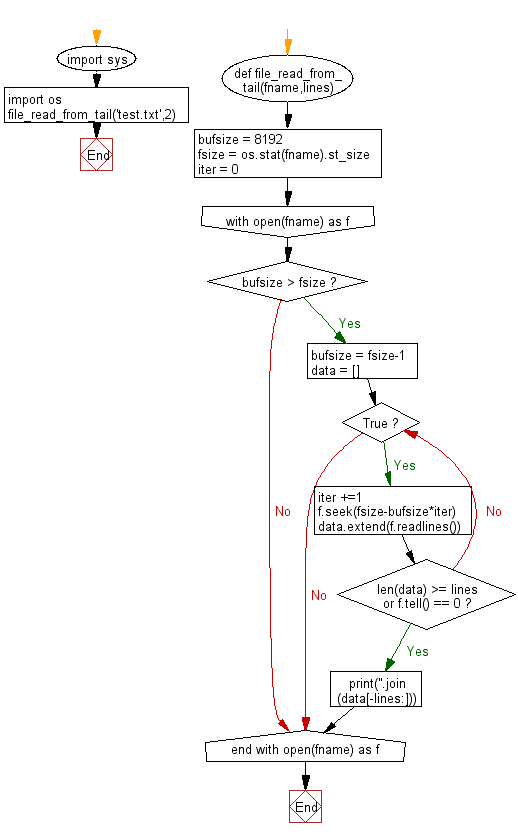
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to append text to a file and display the text.
Next: Write a Python program to read a file line by line and store it into a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics