Python NamedTuple example: Circle attributes
7. Circle NamedTuple
Write a Python program that defines a NamedTuple named "Circle" with fields 'radius' and 'center' (a Point NamedTuple representing the coordinates of the center of the circle). Create an instance of the "Circle" NamedTuple and print its attributes
Sample Solution:
Code:
from collections import namedtuple
# Define a Point NamedTuple
Point = namedtuple('Point', ['x', 'y'])
# Define a Circle NamedTuple
Circle = namedtuple('Circle', ['radius', 'center'])
# Create an instance of the Circle NamedTuple
center = Point(x=4, y=4) # Center at (0, 0)
circle_instance = Circle(radius=5, center=center)
# Print the attributes of the Circle instance
print("Circle-")
print("Radius:", circle_instance.radius)
print("Center:", circle_instance.center)
print("Value of x-coordinate:", circle_instance.center.x)
print("Value of y-coordinate", circle_instance.center.y)
Output:
Circle- Radius: 5 Center: Point(x=4, y=4) Value of x-coordinate: 4 Value of y-coordinate 4
In the exercise above, we declare a "Point" NamedTuple to represent the coordinates of a point. Next, we define a "Circle" NamedTuple with 'radius' and 'center' fields, where 'center' is an instance of the "Point" NamedTuple. Finally, we create a "Circle" NamedTuple instance and print its attributes, including the radius and the center.
Flowchart:
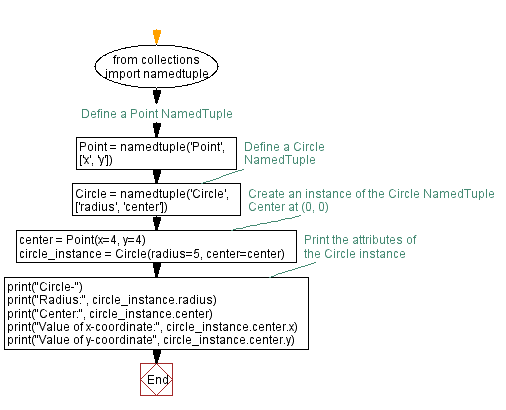
For more Practice: Solve these Related Problems:
- Write a Python program to define a NamedTuple 'Circle' with fields: radius and center (a `Point` NamedTuple), then compute and print the circle’s circumference.
- Write a Python function that creates a 'Circle' NamedTuple and calculates its area using the radius.
- Write a Python script to compare two 'Circle' NamedTuples based on their areas and print the one with the larger area.
- Write a Python program to modify a 'Circle' NamedTuple by updating its center and then print the updated circle's attributes.
Go to:
Previous: Python NamedTuple function: Calculate average grade.
Next: Python NamedTuple example: Triangle area calculation.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.