Python NamedTuple function: Calculate average grade
Python NamedTuple Data Type: Exercise-6 with Solution
Write a Python function that takes a Student named tuple as an argument and calculates the average grade.
Sample Solution:
Code:
from collections import namedtuple
# Define a NamedTuple named 'Student' with fields 'name', 'age', and 'marks'
Student = namedtuple("Student", ["name", "age", "marks"])
def calculate_average_grade(student):
total_marks = sum(student.marks)
average_grade = total_marks / len(student.marks)
return average_grade
# Create an instance of the Student NamedTuple
student1 = Student(name="Ain Ruth", age=22, marks=[89, 92, 75, 90, 86])
# Calculate and print the average grade for the student
average_grade = calculate_average_grade(student1)
print("Average Grade:", average_grade)
Output:
Average Grade: 86.4
In the exercise above, we declare a "Student" NamedTuple with the fields 'name', 'age', and 'marks'. The "calculate_average_grade()" function takes a "Student" NamedTuple as input, calculates the average grade from the 'marks' field, and returns it. Next, we create an instance of the "Student" NamedTuple and calculate and print the average grade.
Flowchart:
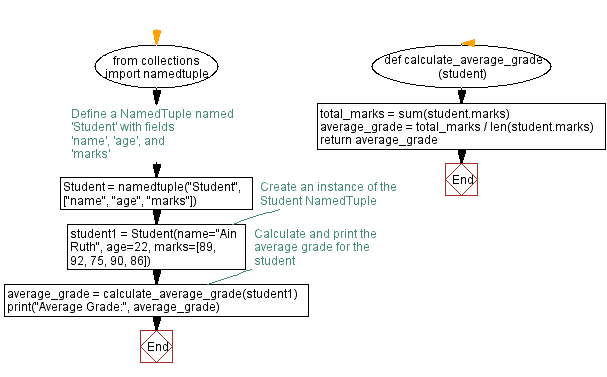
Previous: Python NamedTuple function example: Get item information.
Next: Python NamedTuple example: Circle attributes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics