Slicing memory views in Python: Indexing syntax and example
Write a Python program that takes a slice of a memory view using indexing syntax and prints the slice.
Sample Solution:
Code:
def main():
original_data = bytearray([1, 2, 3, 4, 5, 6, 7, 8, 9, 10])
memory_view = memoryview(original_data)
# Taking a slice of the memory view
start_index = 3
end_index = 8
sliced_memory_view = memory_view[start_index:end_index]
print("Original Data:", original_data)
print("Sliced Memory View:")
print(bytearray(sliced_memory_view))
print(sliced_memory_view.tolist())
if __name__ == "__main__":
main()
Output:
Original Data: bytearray(b'\x01\x02\x03\x04\x05\x06\x07\x08\t\n') Sliced Memory View: bytearray(b'\x04\x05\x06\x07\x08') [4, 5, 6, 7, 8]
In the exercise above, the "main()" function creates a bytearray and converts it to a memory view. Then, it uses indexing syntax to take a slice of the memory view, from index 3 (inclusive) to index 8 (exclusive). Using the tolist() method, the sliced memory view is converted to a list.
Flowchart:
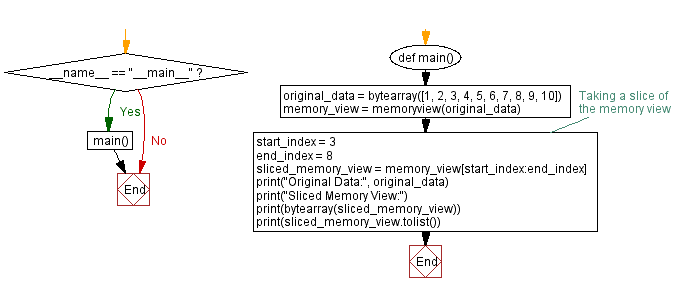
Previous: Reversing memory views in Python: Example and steps .
Next: Hexadecimal values of list elements using memory views in Python.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics