Python program: Generating power set of a frozenset
Python Frozenset Views Data Type: Exercise-4 with Solution
Write a Python program that implements a function to generate the power set (set of all subsets) of a given frozenset.
Sample Solution:
Code:
def powerset(frozenset_input):
power_set = [[]]
for element in frozenset_input:
new_subsets = []
for subset in power_set:
new_subset = subset + [element]
new_subsets.append(new_subset)
power_set.extend(new_subsets)
return [frozenset(subset) for subset in power_set]
def main():
frozen_set = frozenset([1, 2, 3, 4])
print("Original Power set")
power_set_result = powerset(frozen_set)
print("Power Set:")
for subset in power_set_result:
print(subset)
if __name__ == "__main__":
main()
Output:
Original Power set Power Set: frozenset() frozenset({1}) frozenset({2}) frozenset({1, 2}) frozenset({3}) frozenset({1, 3}) frozenset({2, 3}) frozenset({1, 2, 3}) frozenset({4}) frozenset({1, 4}) frozenset({2, 4}) frozenset({1, 2, 4}) frozenset({3, 4}) frozenset({1, 3, 4}) frozenset({2, 3, 4}) frozenset({1, 2, 3, 4})
Flowchart:
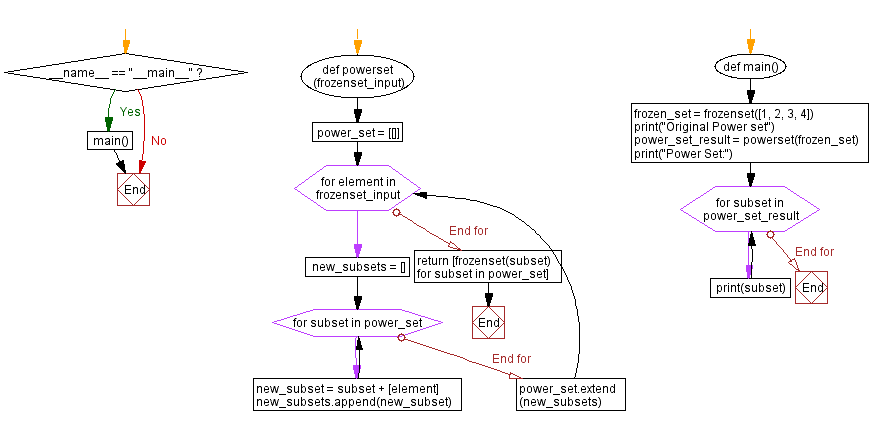
Previous: Python frozenset symmetric difference: Function and example.
Next: Python power set generator for frozensets: Code and example.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics