Python Function: Calling functions with flexible arguments
Write a Python function that takes a function as an argument and calls it with any number of arguments.
Sample Solution:
Code:
def call_function(func, *args, **kwargs):
"""
Calls the given function with any number of positional and keyword arguments.
Args:
func (function): The function to call.
*args: Any positional arguments to pass to the function.
**kwargs: Any keyword arguments to pass to the function.
Returns:
The result of calling the function.
"""
return func(*args, **kwargs)
# Example usage:
def add(x, y):
return x + y
result = call_function(add, 10, 22)
print(result) # Output: 8
def message(name, text="Good Evening"):
return f"{name}, {text}!"
result = call_function(message, "Erich", text = ...)
print(result)
Output:
32 Erich, Ellipsis!
In the exercise above, the "call_function()" function takes a 'func' argument, which is the function to call, and uses *args and **kwargs to accept any number of positional and keyword arguments. It then calls 'func' with these arguments and returns the result. As a result, you can call different functions with different numbers of arguments by using the same "call_function ()".
In this code, the ellipsis (...) is used as a placeholder for unspecified arguments in the function calls 'add' and 'message'. It indicates that there can be additional positional or keyword arguments.
Flowchart:
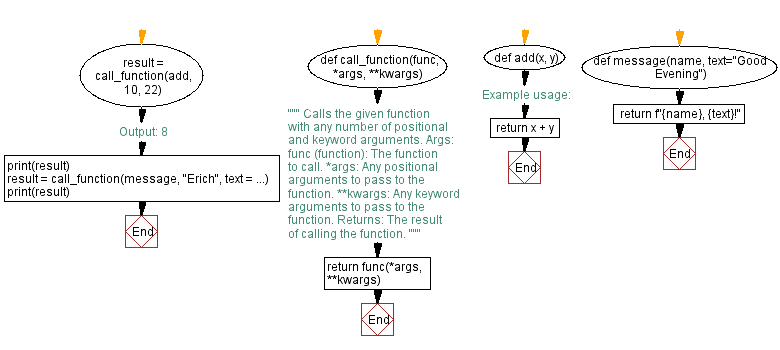
Previous: Python Function: Printing arguments with *args.
Next: Python Function: Slicing the third dimension of a multidimensional array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics