Python counter filter program: Counting and filtering words
8. Filter Counter by Threshold
Write a Python program that creates a 'Counter' for a list of words and removes all items with a count less than a certain value.
Sample Solution:
Code:
from collections import Counter
def main():
colors = ["Red", "Green", "Black", "Black", "Red", "red", "Orange", "Pink", "Pink", "Red", "White"]
color_counter = Counter(colors)
min_count = 2
filtered_counter = {word: count for word, count in color_counter.items() if count >= min_count}
print("Original List:", colors)
print("Counter:", color_counter)
print(f"Items with Count >= {min_count}:", filtered_counter)
if __name__ == "__main__":
main()
Output:
Original List: ['Red', 'Green', 'Black', 'Black', 'Red', 'red', 'Orange', 'Pink', 'Pink', 'Red', 'White'] Counter: Counter({'Red': 3, 'Black': 2, 'Pink': 2, 'Green': 1, 'red': 1, 'Orange': 1, 'White': 1}) Items with Count >= 2: {'Red': 3, 'Black': 2, 'Pink': 2}
In the exercise above, the "Counter" class is used to create a counter for the list of words. The 'filtered_counter' is then created by filtering out items with a count less than the specified 'min_count' from the original counter. Finally results are displayed, including the original list, the counter, and filtered items exceeding or equal to 'min_count'.
Flowchart:
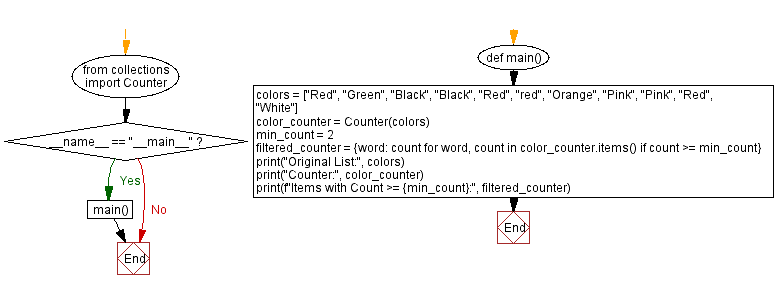
For more Practice: Solve these Related Problems:
- Write a Python program to create a Counter from a list of words and remove all entries with a count less than 2, then print the filtered Counter.
- Write a Python script to filter a Counter by deleting items with counts below a user-defined threshold and then output the updated Counter.
- Write a Python function that takes a list and a threshold value, builds a Counter, and returns a new Counter with only keys having counts above the threshold.
- Write a Python program to filter a Counter’s keys by a minimum count value and then display the remaining items sorted by frequency.
Go to:
Previous: Python Program: Convert counter to list of unique Items.
Next: Python Program: Calculate Jaccard Similarity Coefficient.
Python Code Editor :
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.