Python Program: Counting and sorting word frequencies
Python Counter Data Type: Exercise-5 with Solution
Write a Python program that creates a counter of the words in a sentence and prints the words in ascending and descending order of their frequency.
Sample Solution:
Code:
from collections import Counter
def main():
sentence = "Red Green Black Black Red red Orange Pink Pink Red White."
# Split the sentence into words
words = sentence.split()
# Create a Counter of the words
word_counter = Counter(words)
# Print words in ascending order of their frequencies
print("Words in Ascending Order:")
sorted_words_asc = sorted(word_counter.items(), key=lambda item: item[1])
for word, count in sorted_words_asc:
print(f"{word}: {count}")
print("\n")
# Print words in descending order of their frequencies
print("Words in Descending Order:")
sorted_words_desc = sorted(word_counter.items(), key=lambda item: item[1], reverse=True)
for word, count in sorted_words_desc:
print(f"{word}: {count}")
if __name__ == "__main__":
main()
Output:
Words in Ascending Order: Green: 1 red: 1 Orange: 1 White.: 1 Black: 2 Pink: 2 Red: 3 Words in Descending Order: Red: 3 Black: 2 Pink: 2 Green: 1 red: 1 Orange: 1 White.: 1
In the exercise above, the words in the sentence are counted using a "Counter" object 'word_counter'. The "sorted()" function sorts words based on their frequencies in both ascending and descending orders. In the "sorted()" function, the 'key' parameter specifies the sorting criteria, and the 'reverse' parameter controls the sorting order. In both ascending and descending order, the program prints the words and their frequencies.
Flowchart:
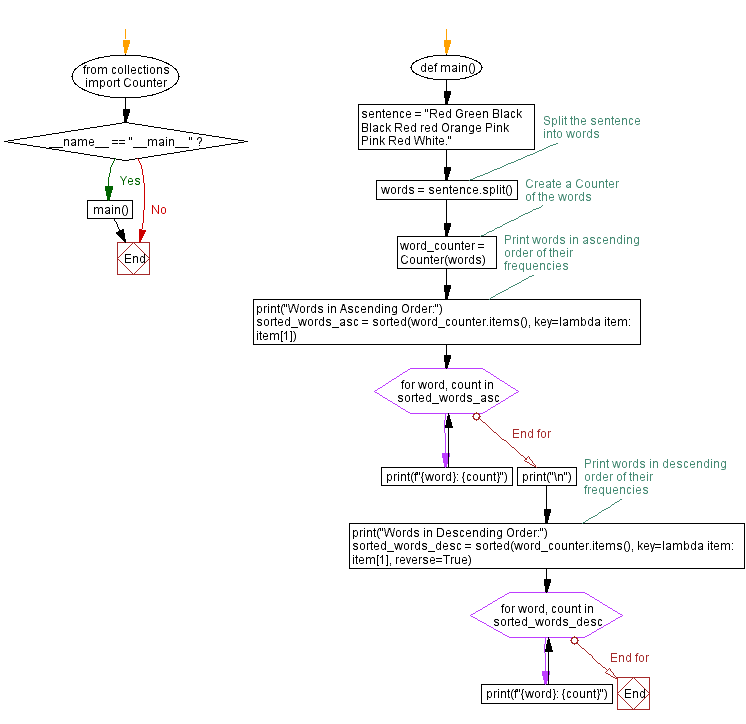
Previous: Python Program: Counter operations - Union, intersection, and difference.
Next: Python Counter sum function: Add two counter objects.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics