Python Program: Counter operations - Union, intersection, and difference
Write a Python program that creates two 'Counter' objects and finds their union, intersection, and difference.
Sample Solution:
Code:
from collections import Counter
# Create two Counter objects
ctr1 = Counter(x=3, y=2, z=10)
ctr2 = Counter(x=1, y=2, z=3)
# Union of counters
union_counter = ctr1 + ctr2
print("Union Counter:", union_counter)
# Intersection of counters
intersection_counter = ctr1 & ctr2
print("\nIntersection Counter:", intersection_counter)
# Difference of counters
difference_counter = ctr1 - ctr2
print("\nDifference Counter:", difference_counter)
Output:
Union Counter: Counter({'z': 13, 'x': 4, 'y': 4}) Intersection Counter: Counter({'z': 3, 'y': 2, 'x': 1}) Difference Counter: Counter({'z': 7, 'x': 2})
In the exercise above, two "Counter" objects 'ctr1' and 'ctr2' are created with different key-value pairs. The union operation '+' adds up the counts of the common keys in both counters. The intersection operation '&' keeps the minimum count of common keys. The difference operation '-' subtracts the count of keys in the second counter from the first counter. Finally each case's results are printed.
Flowchart:
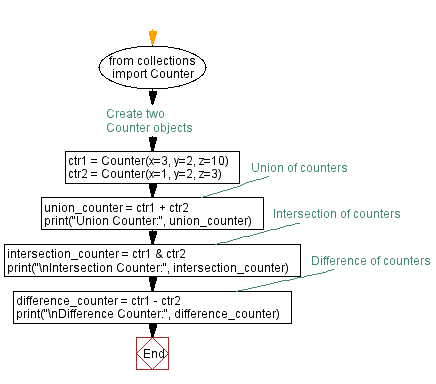
Previous: Python Program: Counting vowels in a word.
Next: Python Program: Counting and sorting word frequencies.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics