Python Program: Counting vowels in a word
Python Counter Data Type: Exercise-3 with Solution
Write a Python program that creates a counter of the vowels in the word "Python Exercises".
Sample Solution:
Code:
from collections import Counter
word = "Python Exercises"
vowels = "aeiouAEIOU" # List of vowels
vowel_ctr = Counter(c for c in word if c in vowels)
print("Vowel Counts:")
for vowel, count in vowel_ctr.items():
print(f"{vowel}: {count}")
Output:
Vowel Counts: o: 1 E: 1 e: 2 i: 1
In the exercise above program, the "Counter" class counts the occurrences of each vowel in the given word. Each character in the word is checked to see if it is a vowel by the program. If it is a vowel, its count is updated in 'vowel_ctr'. Finally, the program prints the vowel counts.
Flowchart:
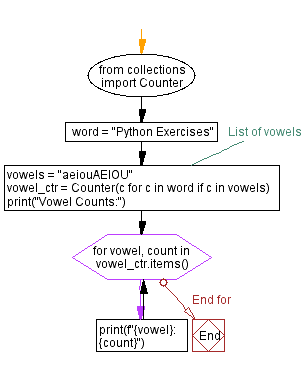
Previous: Python Program: Counting common elements in a list.
Next: Python Program: Counter operations - Union, intersection, and difference.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/extended-data-types/python-extended-data-types-index-counter-exercise-3.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics