Python Program: Updating item counts using Counter objects
10. Update Counter via Dictionary Access
Write a Python program that creates a 'Counter' for a list of items and uses dictionary-style access to update the count of specific items.
Sample Solution:
Code:
from collections import Counter
def main():
items = ['Red', 'Green', 'Black', 'Black', 'Red', 'Red', 'Orange', 'Pink', 'Pink', 'Red', 'White']
# Create a Counter for the list of items
counter = Counter(items)
print("Original Counter:")
print(counter)
# Update the count of specific items using dictionary-style access
counter['Red'] += 2
counter['Orange'] -= 1
print("\nUpdated Counter:")
print(counter)
if __name__ == "__main__":
main()
Output:
Original Counter: Counter({'Red': 4, 'Black': 2, 'Pink': 2, 'Green': 1, 'Orange': 1, 'White': 1}) Updated Counter: Counter({'Red': 6, 'Black': 2, 'Pink': 2, 'Green': 1, 'White': 1, 'Orange': 0})
In the exercise above, the "Counter" is first created for the list of items. The count of specific items is then updated using dictionary-style access, where we provide the item as the key and the new count as the value. In order to show the changes in the counts after updating, the modified "Counter" is printed.
Flowchart:
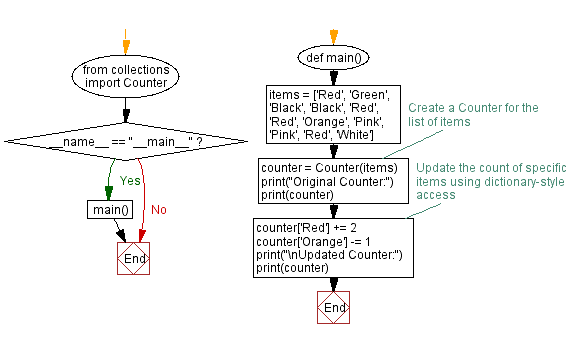
For more Practice: Solve these Related Problems:
- Write a Python program to create a Counter from a list, then update the count of specific items using dictionary-style access, and print the modified Counter.
- Write a Python script to manually increment the counts of certain keys in a Counter and verify the updates by comparing before and after values.
- Write a Python function that takes a Counter and a mapping of updates, applies the updates using dict-style assignment, and returns the updated Counter.
- Write a Python program to create a Counter, update the count for a given key directly, and then check if the updated count matches the expected value.
Python Code Editor :
Previous: Python Program: Calculate Jaccard Similarity Coefficient.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.