Python Program: Counting letters in a string
Write a Python program to create a 'Counter' of the letters in the string "Python Exercise!".
Sample Solution:
Code:
from collections import Counter
text = "Python Exercise!"
letter_counter = Counter(text)
print("Letter Counter:")
for letter, count in letter_counter.items():
if letter.isalpha():
print(f"{letter}: {count}")
Output:
Letter Counter: P: 1 y: 1 t: 1 h: 1 o: 1 n: 1 E: 1 x: 1 e: 2 r: 1 c: 1 i: 1 s: 1
In the exercise above "Counter" class from the "collections" module to count the occurrences of each letter in the given string. It then iterates through the items in the "Counter" and prints the counts of letters that are alphabetic characters.
Flowchart:
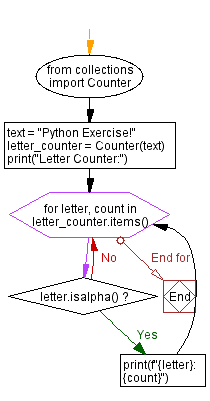
Previous: Python Extended Data Type Counter Exercises Home.
Next: Python Program: Counting common elements in a list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics