Python password validation with Boolean expressions
7. Password Validator
Write a Python program that implements a program that validates a user's password based on certain criteria (length, containing numbers and special characters etc.) using boolean expressions.
Sample Solution:
Code:
def Is_password_valid(password):
# Check if password length is between 8 and 16 characters
if len(password) < 8 or len(password) > 16:
return False
# Check if password contains at least one digit
password_has_digit = any(char.isdigit() for char in password)
# Check if password contains at least one special character
password_special_characters = "!@#$%^&*()_+[]{}|;:,.<>?/"
has_special_character = any(char in password_special_characters for char in password)
# Check if password contains both uppercase and lowercase letters
password_has_uppercase = any(char.isupper() for char in password)
password_has_lowercase = any(char.islower() for char in password)
# Check if all conditions are met
return password_has_digit and has_special_character and password_has_uppercase and password_has_lowercase
def main():
try:
password = input("Input your password: ")
if Is_password_valid(password):
print("Password is valid.")
else:
print("Password is invalid.")
except Exception as e:
print("An error occurred:", e)
if __name__ == "__main__":
main()
Output:
Input your password: afr12@hK$ Password is valid.
Input your password: AQbg12Fert Password is invalid.
This program prompts the user to enter a password and then uses the "Is_password_valid()" function to verify if the password meets the specified criteria. Besides checking the password length, it checks whether it contains at least one digit, special character, uppercase letter, and lowercase letter. It prints the appropriate message based on the results.
Flowchart:
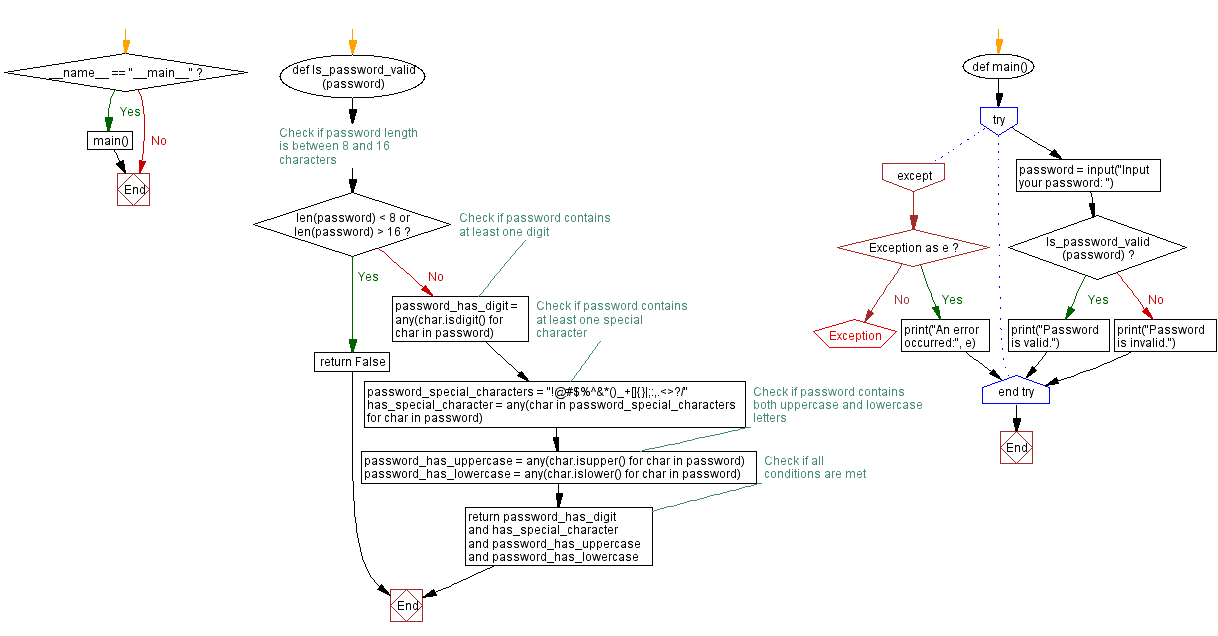
For more Practice: Solve these Related Problems:
- Write a Python program to validate a password by ensuring it meets criteria such as minimum length, inclusion of digits, and special characters using boolean conditions.
- Write a Python function to check a password against multiple boolean conditions (length, uppercase, lowercase, digit, special character) and return a detailed report.
- Write a Python script to prompt the user for a password, validate it using boolean expressions, and output which criteria it failed.
- Write a Python program to implement a password checker that uses boolean flags for each requirement and prints "Valid" only if all conditions are True.
Python Code Editor :
Previous:Python palindrome checker with Boolean logic.
Next: Python email validation using Boolean logic.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.