Python: Find the key of the maximum, minimum value in a dictionary
80. Find Key of Maximum Value in a Dictionary
Write a Python program to find the key of the maximum value in a dictionary.
- Use max() with the key parameter set to dict.get() to find and return the key of the maximum value in the given dictionary.
- Use min() with the key parameter set to dict.get() to find and return the key of the minimum value in the given dictionary.
Sample Solution:
Python Code:
# Define a function 'test' that takes a dictionary 'd'.
# The function returns the keys of the maximum and minimum values in the dictionary.
def test(d):
# Use the 'max' and 'min' functions to find the key corresponding to the maximum and minimum values in the dictionary.
# The 'key' argument specifies that the key should be determined based on the values using 'd.get'.
return max(d, key=d.get), min(d, key=d.get)
# Create a dictionary 'students' with key-value pairs.
students = {
'Theodore': 19,
'Roxanne': 22,
'Mathew': 21,
'Betty': 20
}
# Print the original dictionary.
print("\nOriginal dictionary elements:")
print(students)
# Call the 'test' function to find the keys of the maximum and minimum values in the 'students' dictionary.
print("\nFinds the key of the maximum and minimum value of the said dictionary:")
print(test(students))
Sample Output:
Original dictionary elements: {'Theodore': 19, 'Roxanne': 22, 'Mathew': 21, 'Betty': 20} Finds the key of the maximum and minimum value of the said dictionary: ('Roxanne', 'Theodore')
Flowchart:
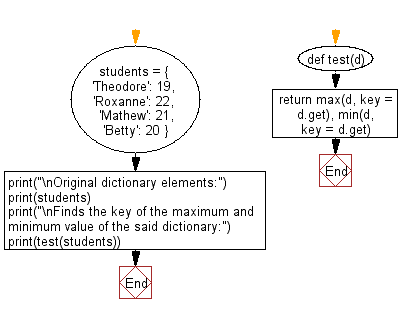
For more Practice: Solve these Related Problems:
- Write a Python program to determine the key associated with the maximum value in a dictionary using max() with a key argument.
- Write a Python program to iterate through a dictionary and return the key for which the value is highest.
- Write a Python program to implement a function that finds the key of the maximum value and returns both the key and value.
- Write a Python program to use dictionary comprehension and the max() function to extract the key with the largest value.
Python Code Editor:
Previous: Write a Python program to create a flat list of all the values in a flat dictionary.
Next: Nested Dictionary Manipulation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.